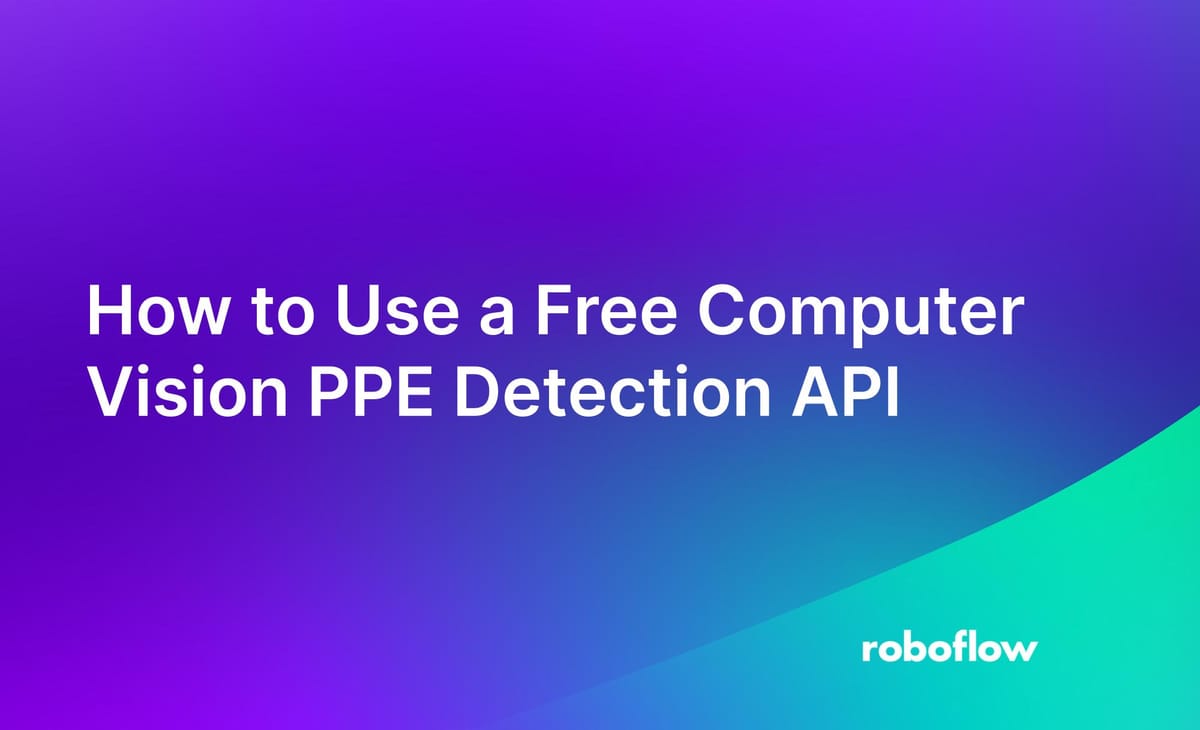
Using computer vision, you can detect the presence or absence of personal protective equipment (PPE) in a video feed.
You can use a PPE detection system powered by computer vision to ensure people are wearing the appropriate PPE in work environments. Such a system helps assure the safety of workers and mitigate the risks associated with not wearing an item of PPE.
Roboflow has a range of custom-trained PPE APIs you can use to detect PPE and instances where someone is not wearing proper PPE (i.e. not wearing a helmet in a construction site). In this guide, we will focus on the Roboflow construction site safety PPE API.
To search for more APIs, check out Roboflow Universe, a community with over 50,000 public computer vision models you can use in your projects.
In this guide, we are going to show how to use the Roboflow construction site safety API to detect PPE. By the end of this guide, we will have predictions from the PPE detection model that we can use to evaluate the presence or absence of PPE in a given environment.
Here is an example of detections from the PPE model plotted on an image:
Without further ado, let’s get started!
Test the PPE Detection API
In this guide, we will use the Construction Site Safety API on Roboflow Universe. This API achieves an 81.4% mAP, 92.7% precision and 77.4% recall.
This API can detect:
- Person
- Safety Cone
- No safety vest
- Hardhat
- No mask
- Safety vest
- No hardhat
- Gloves
- Mask
The model is trained to identify other classes such as vehicle and excavator, but these are underrepresented. You could create a copy of the dataset used to train the underlying model and add additional images to improve the class balance if you need to identify another class in the dataset that is not listed above.
To test the API, go to the Model tab associated with the model in Roboflow Universe. Here, you can test model served by the Construction Site Safety API on images and videos.
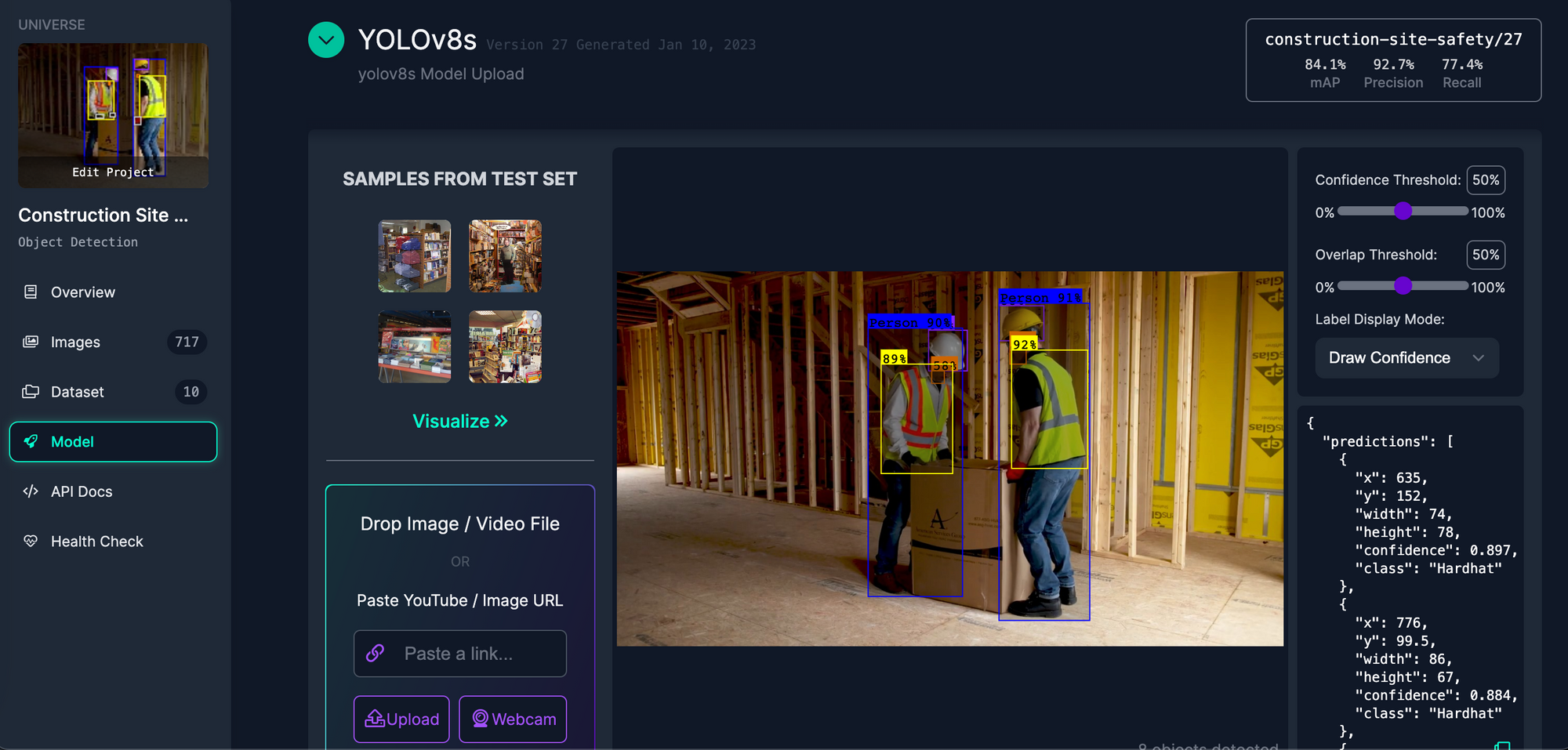
Once you have tested the API, you are ready to start using it in code!
Use the PPE Detection API
Roboflow provides an extensive range of methods for deploying computer vision models, including:
- Luxonis OAK;
- Raspberry Pi;
- NVIDIA Jetson;
- A Docker container;
- iOS;
- A web page and;
- A Python script using the Roboflow SDK.
In this guide, we are going to focus on the Roboflow Python SDK deployment method. To learn more about different deployment methods, follow the instructions associated with each deployment device linked above.
Go to the Model tab associated with the project and scroll down until you see the “Infer on Local and Hosted Images" tab. Copy the code snippet into a new Python file. The snippet will look something like the code below, except your API key will be filled in:
from roboflow import Roboflow
rf = Roboflow(api_key="API_KEY")
project = rf.workspace().project("aquarium-combined")
model = project.version(5).model
# infer on a local image
print(model.predict("your_image.jpg", confidence=40, overlap=30).json())
# visualize your prediction
model.predict("your_image.jpg", confidence=40, overlap=30).save("prediction.jpg")
Update the “your_image.jpg” value with the name of the image on which you want to run inference. Then, run the script.
The raw predictions data will be printed to the console:
{'predictions': [{'x': 389.5, 'y': 197.5, 'width': 175.0, 'height': 163.0, 'confidence': 0.9049781560897827, 'class': 'Hardhat', 'class_id': 0, 'image_path': 'your_image.jpg', 'prediction_type': 'ObjectDetectionModel'}, {'x': 376.0, 'y': 311.5, 'width': 108.0, 'height': 73.0, 'confidence': 0.8808342218399048, 'class': 'NO-Mask', 'class_id': 3, 'image_path': 'your_image.jpg', 'prediction_type': 'ObjectDetectionModel'}, {'x': 377.5, 'y': 372.0, 'width': 497.0, 'height': 532.0, 'confidence': 0.8804072141647339, 'class': 'Person', 'class_id': 5, 'image_path': 'your_image.jpg', 'prediction_type': 'ObjectDetectionModel'}, {'x': 365.5, 'y': 380.0, 'width': 223.0, 'height': 260.0, 'confidence': 0.6987550258636475, 'class': 'NO-Safety Vest', 'class_id': 4, 'image_path': 'your_image.jpg', 'prediction_type': 'ObjectDetectionModel'}], 'image': {'width': '640', 'height': '640'}}
You will see predictions from the model plotted on an image saved to a file called “prediction.jpg”:
Connecting PPE Detection with Business Logic
Now that you can retrieve predictions, you can start writing logic for a PPE detection API. For that, we recommend supervision, an open source Python package that provides a range of utilities for use in building computer vision applications. supervision is actively maintained by the Roboflow team.
With supervision, you can:
- Filter predictions by class, box area, confidence, and more.
- Plot object detection and segmentation predictions on an image.
- Use ByteTrack for object tracking.
- Use SAHI for small object detection.
- And more.
To see the full range of capabilities available in supervision, read the supervision documentation.
Conclusion
In this guide, we showed how to use a Roboflow PPE detection API. The API we showed allows you to identify the presence or absence of various items of PPE such as gloves and masks.
We showed how to test the model in the Roboflow Deploy tab on Universe, then we used the Python SDK to run the model in code. We plotted predictions onto an image and retrieved a JSON representation of the predictions.
Cite this Post
Use the following entry to cite this post in your research:
James Gallagher. (Oct 6, 2023). How to Use a Free Computer Vision PPE Detection API. Roboflow Blog: https://blog.roboflow.com/ppe-detection-api/
Discuss this Post
If you have any questions about this blog post, start a discussion on the Roboflow Forum.