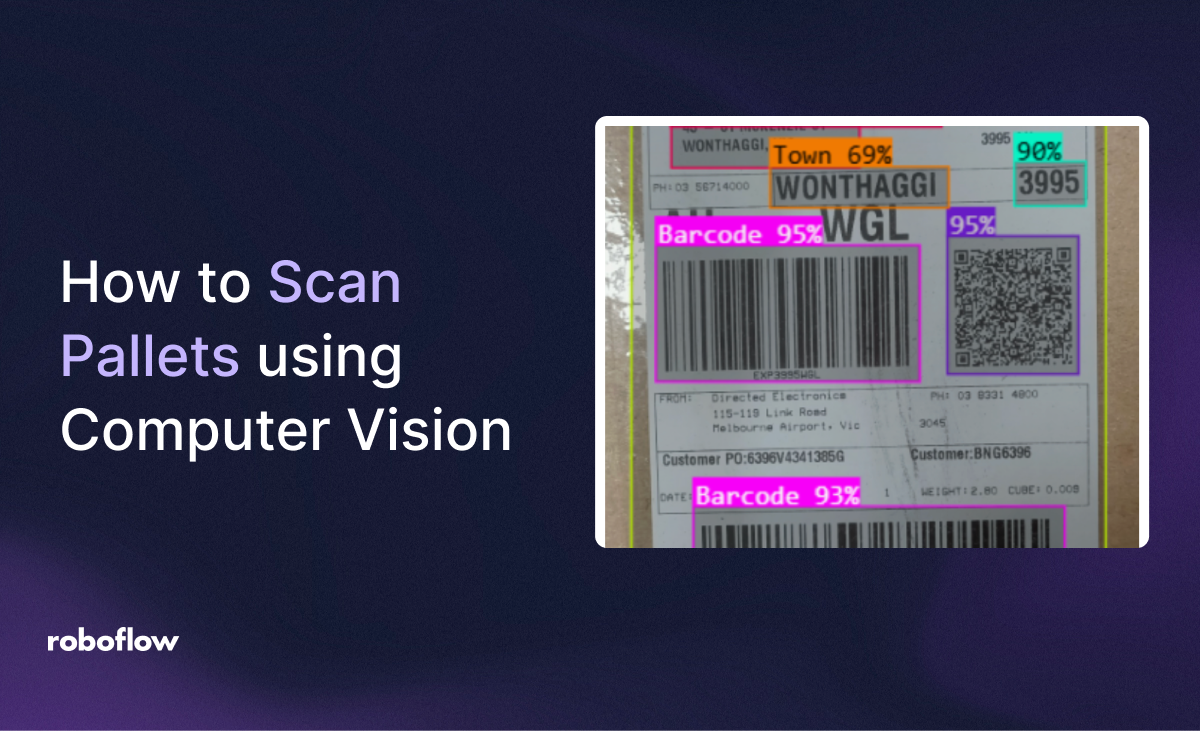
Pallet scanning using computer vision is the process to detect, identify, and interpret information from pallets labels in industrial settings, such as warehouses, distribution centers, or manufacturing facilities.
This process typically involves capturing visual data (e.g., barcodes, labels, or QR codes etc.) using cameras or sensors and analyzing it with computer vision algorithms to automate tasks like inventory tracking, pallet movement, and logistics management.
In pallet scanning, specific types of information and "documents" on pallets are scanned. Here, "documents" typically refer to labels, tags, or markings that provide critical data about the pallet and its contents. There can be the following type of information which is scanned.
- Barcodes and QR Codes: It contains key data such as product identification numbers, batch or lot codes, and shipping details. They are central to automating tracking and inventory management.
- Printed Text and Labels: In addition to barcodes, pallets often have printed text that may include product names, handling instructions, or destination details. Optical Character Recognition (OCR) is used to extract this text data.
- Logistics Information: This might include information about the shipment, such as delivery dates, routing numbers, or other tracking identifiers embedded in the labels. Sometimes, even additional documentation attached to the pallet (e.g., shipping manifests or packing lists) may be scanned if integrated into the process.
Here is an example result from the application we will build in this guide:
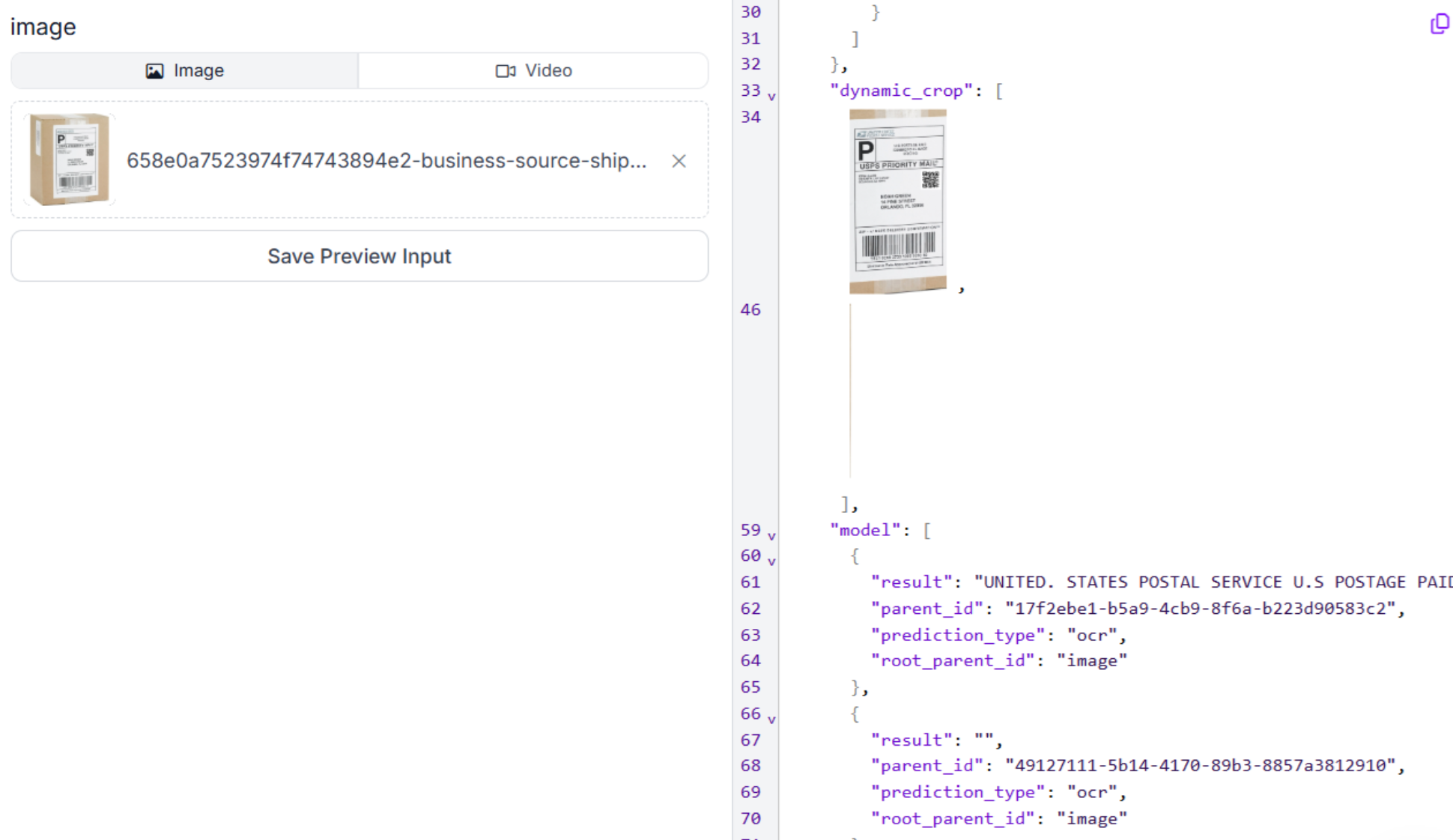
Our application successfully identifies the shipping label on a box and reads the text on it.
How Pallet Scanning Works with Computer Vision
Pallet scanning using computer vision involves several key steps to automate the identification and processing of pallets in industrial settings. Here's a detailed breakdown of each step:
Image Capture
High-resolution cameras take clear pictures of pallet labels. Cameras might be positioned to capture multiple sides of the pallet for better accuracy.
Label Detection
The captured images are analyzed to find labels, either by using traditional computer vision techniques like edge detection or pattern recognition methods or deep learning methods. Labels can be identified even if they're placed differently on each pallet.
Decoding Information
If labels contain barcodes or QR codes, the system uses decoding algorithms to extract data. If labels contain text, optical character recognition (OCR) reads and extracts the text.
Data Integration
The decoded information (like product IDs or tracking numbers) is sent to the warehouse management system. This data helps track pallets, manage inventory, and automate logistics processes.
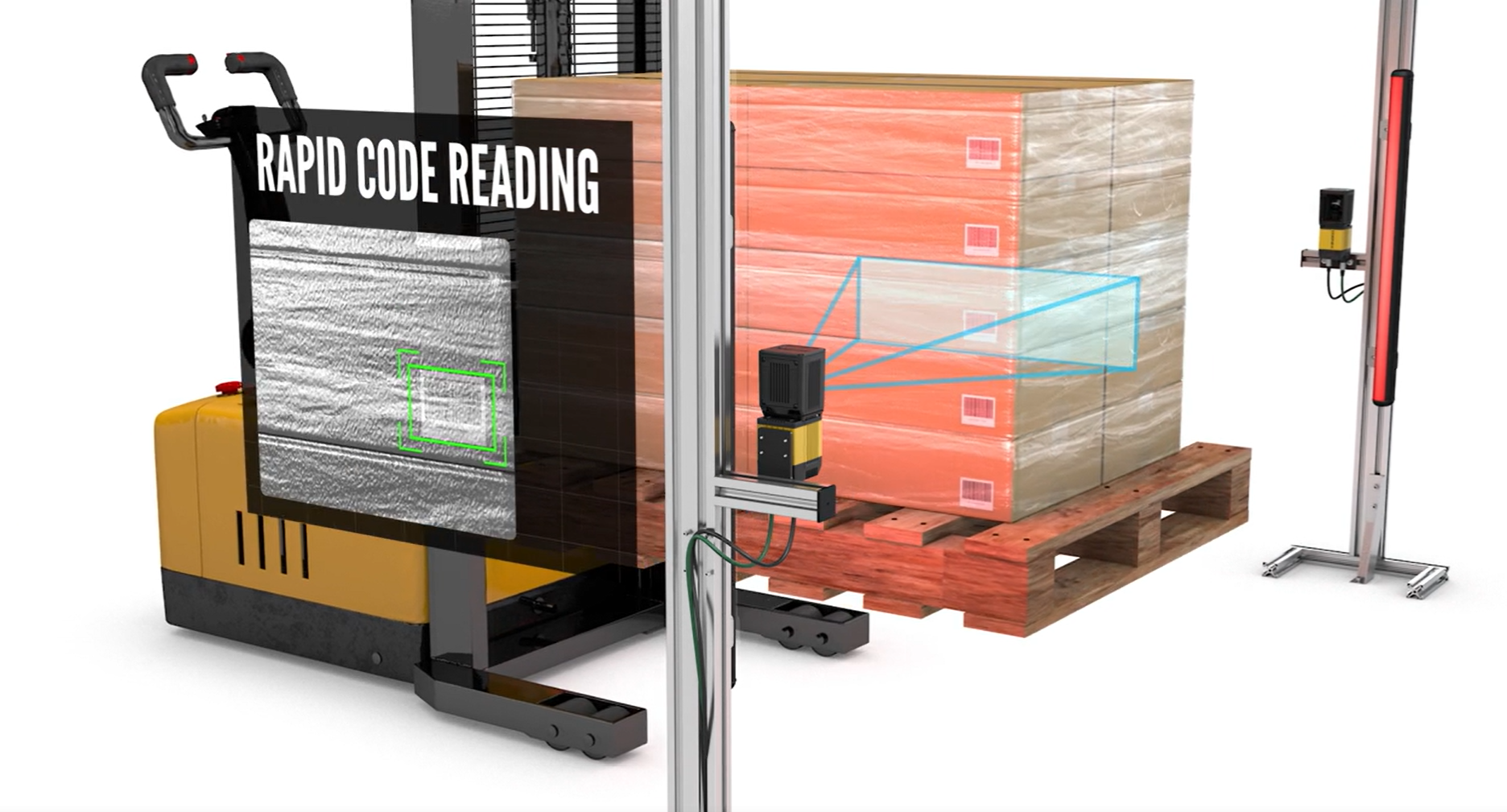
How to Build a Pallet Scanning Application
Now we will see how to build a pallet scanning application using computer vision. We will use Roboflow workflows for building the application. We will learn to build applications for the following three tasks:
- Detect and read barcode on pallet
- Detect and read QR code on pallet
- Detect labels and read OCR
The following are the steps for building the application.
Step #1: Train a computer vision model
First, train a computer vision model to detect the label, barcode, QR code or any other relevant information on the document on pallets. I build a computer vision model and label the dataset with following classes.
- Address
- Barcode
- QR code
- Post Code
- Town
- Shipping-Label
The following image shows how the images are labeled.
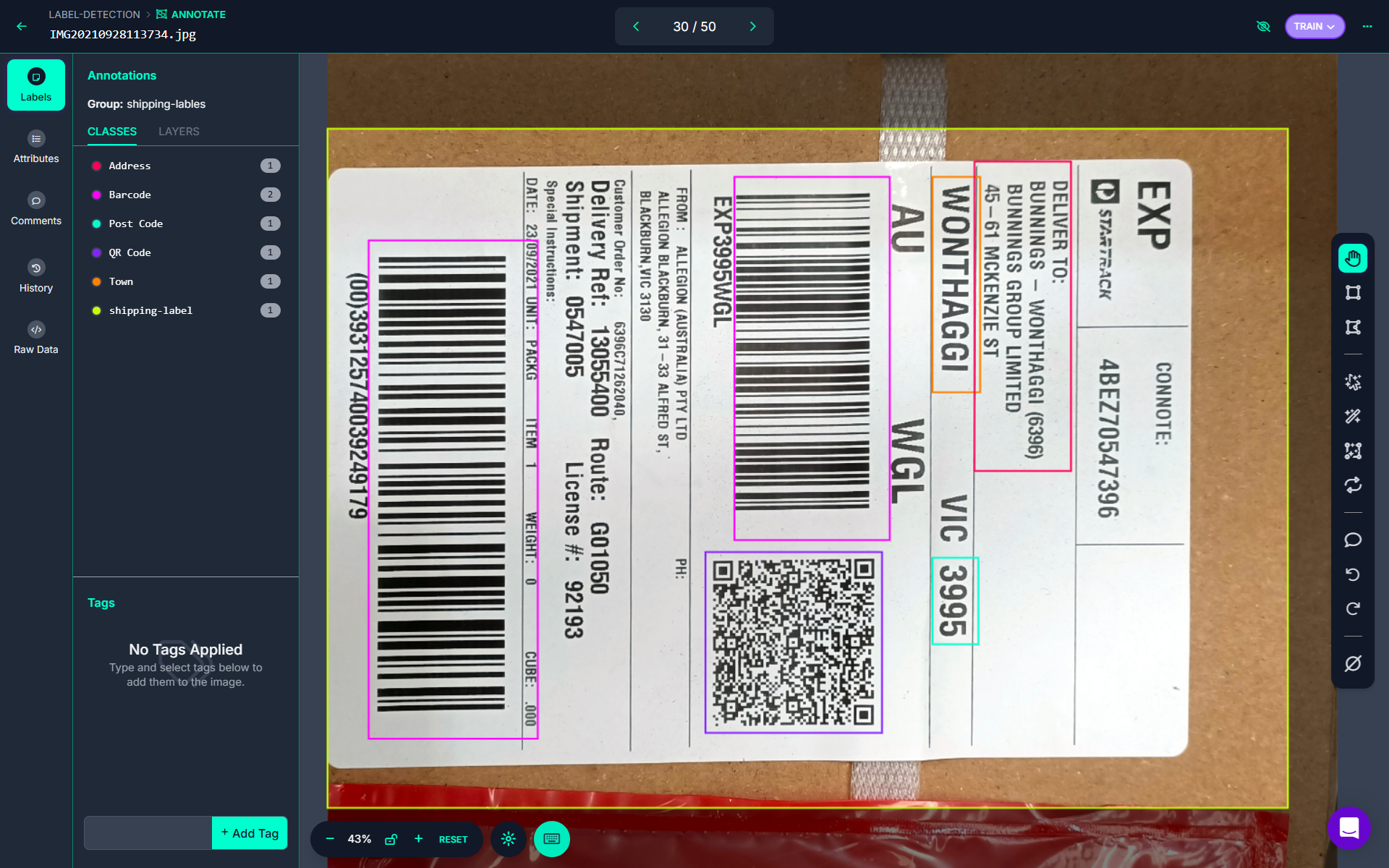
The model is trained using Roboflow's auto-training feature. As shown in the image below, it effectively identifies each class with high accuracy.
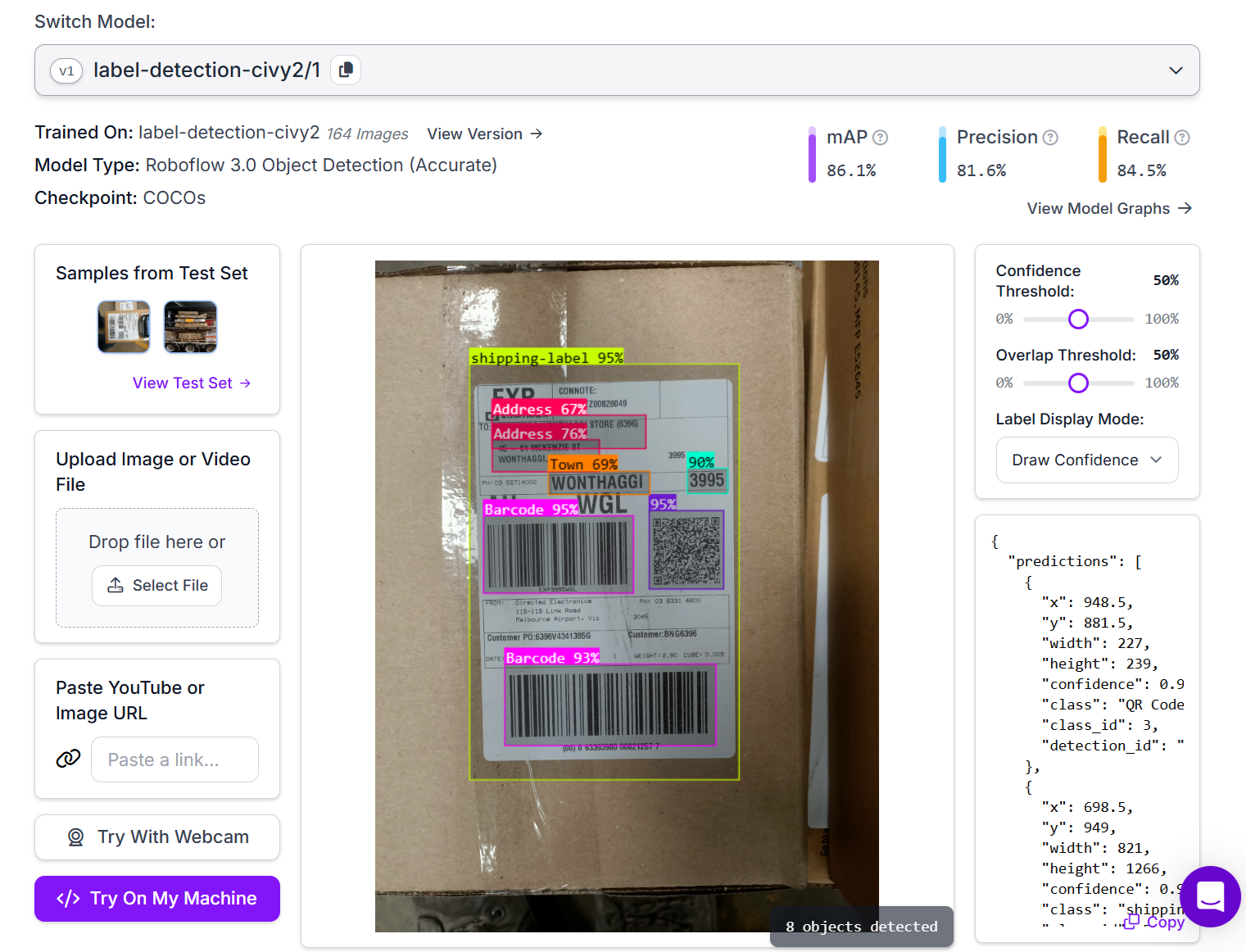
Using this model, we will develop an application for the pallet scanning process.
Step #2: Build the pallet scanning application
In this step, we will learn how to easily build applications using the Roboflow workflow. We will create separate workflows for three tasks, barcode reading, QR code reading, and OCR which are essential components of pallet scanning. You have the option to build individual workflows for each task or combine them into a single workflow; here, we will build separate workflows for each.
Step #2.1: Reading barcode
Create the workflow for detecting and reading barcode as following. The workflow has Object Detection Model block, Dynamic Crop block, Barcode detection block along with the input and output block.
The Barcode detection block is designed to both locate and read barcodes within an image. However, in our example, an object detection model identifies the barcode, and then a dynamic crop is applied to isolate the barcode area from the rest of the image. This ensures that only the relevant barcode data is processed, which reduces interference time and speeding up the detection of multiple barcodes.
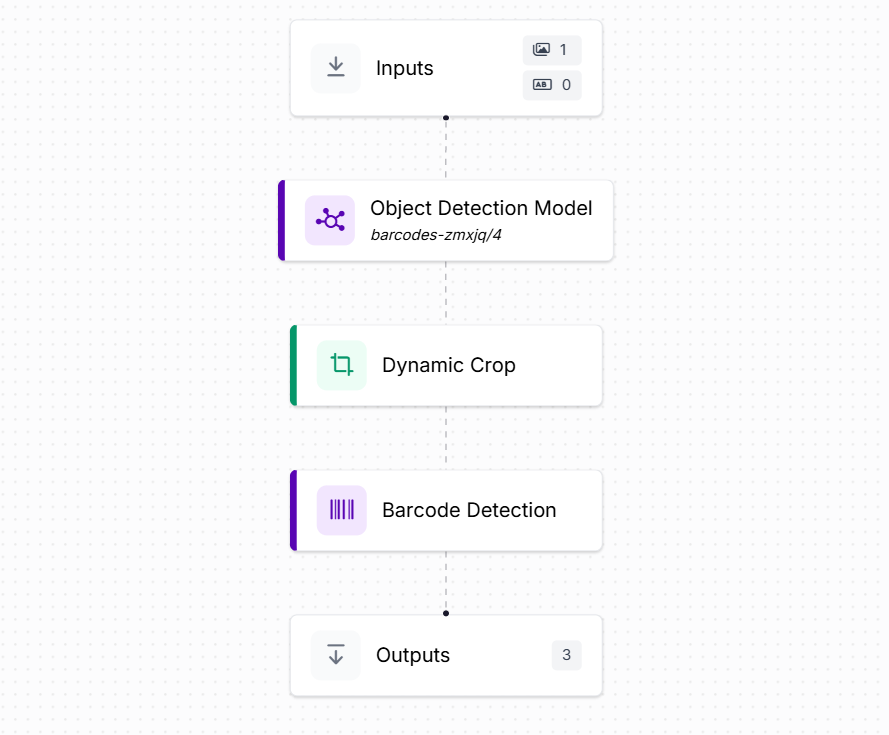
In the workflow above, select your barcode detection computer vision model for the object detection block. If the model outputs multiple classes, specify the barcode class in the Class Filter so that only barcode detections are returned. For instance, if the model built in step 1 detects several classes including "Barcode," setting the Class Filter to "Barcode" ensures that only that class is used for barcode detection.
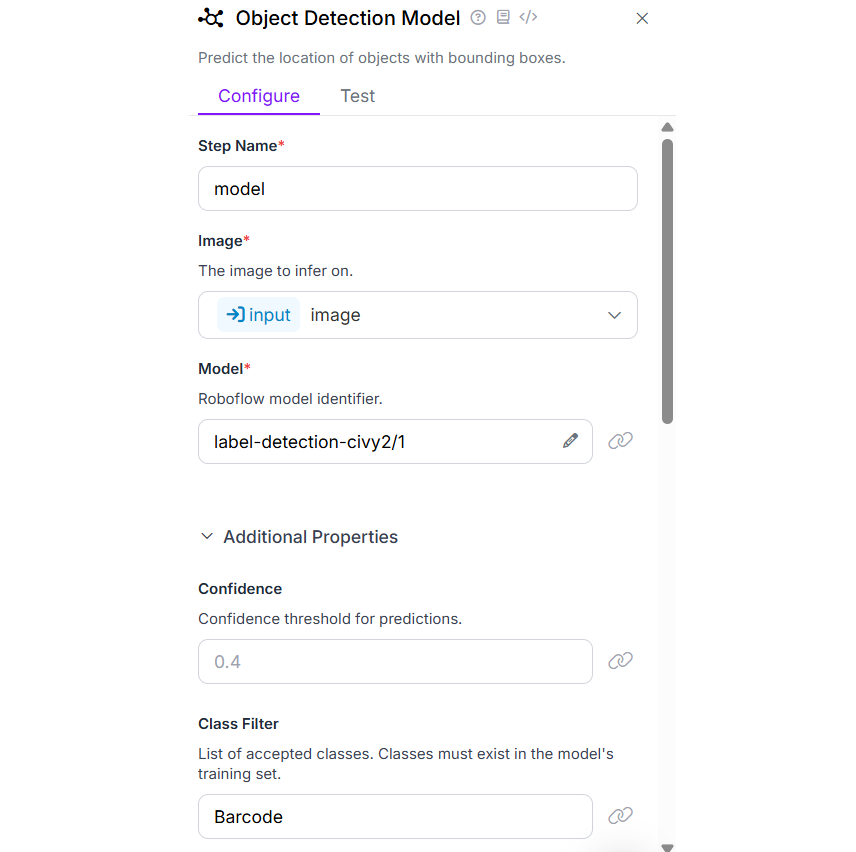
When you run the workflow and upload an image with a barcode, it will decode the barcode and return the information, which can then be utilized further. You will see the barcode data as follows:
"data": "(91)21026837331000001010"
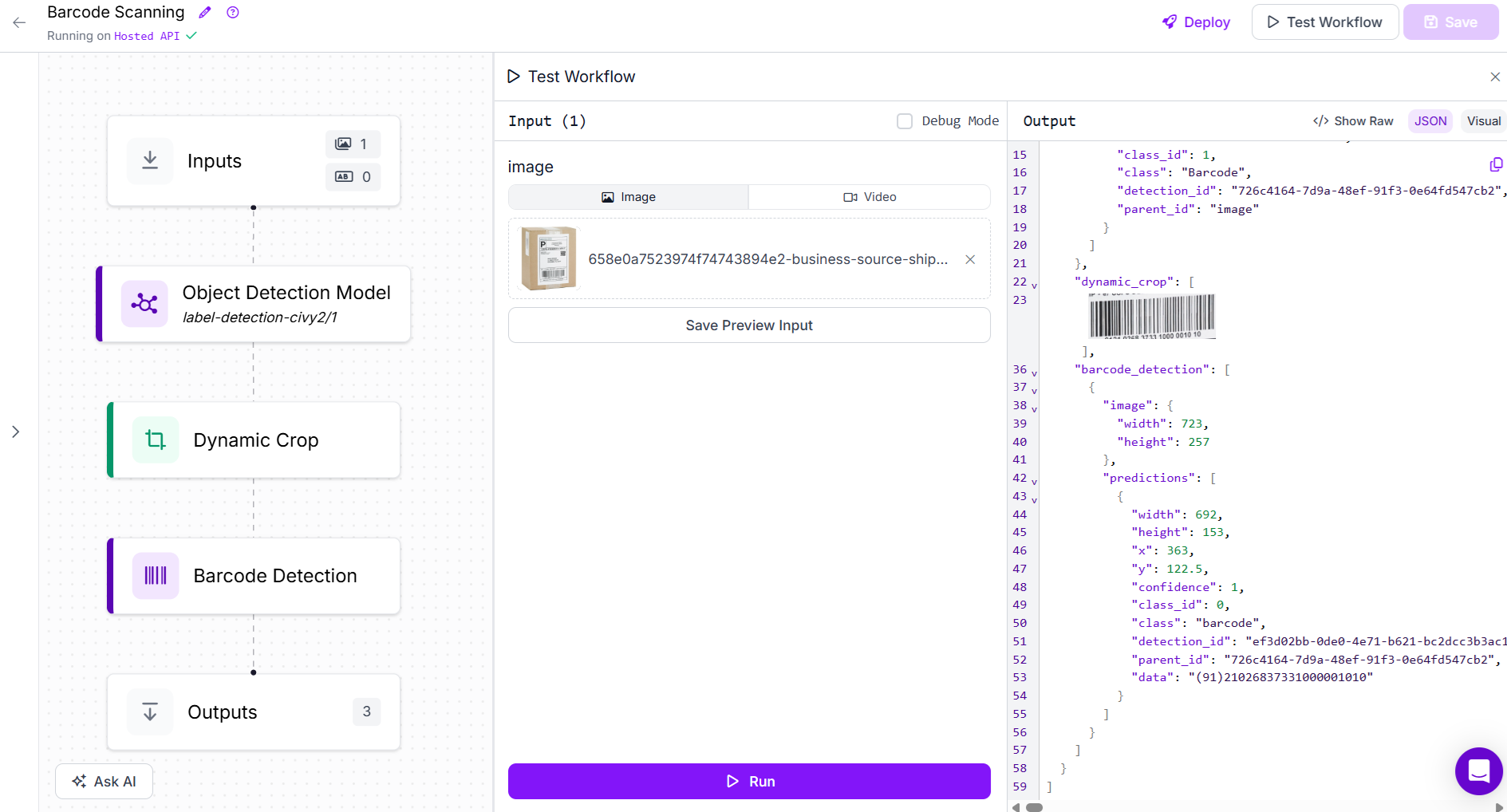
Step #2.2: Reading QR code
Create a workflow similar to step 2.1 but with QR code detection block instead of barcode detection block. The QR code block here detects and reads the QR code.
The QR Code detection block identifies and also reads QR codes within an image. however, in this example an object detection model is used to locate the QR code, after which a dynamic crop is applied to isolate the QR code region from the rest of the image. This speeds up multiple QR codes detection.
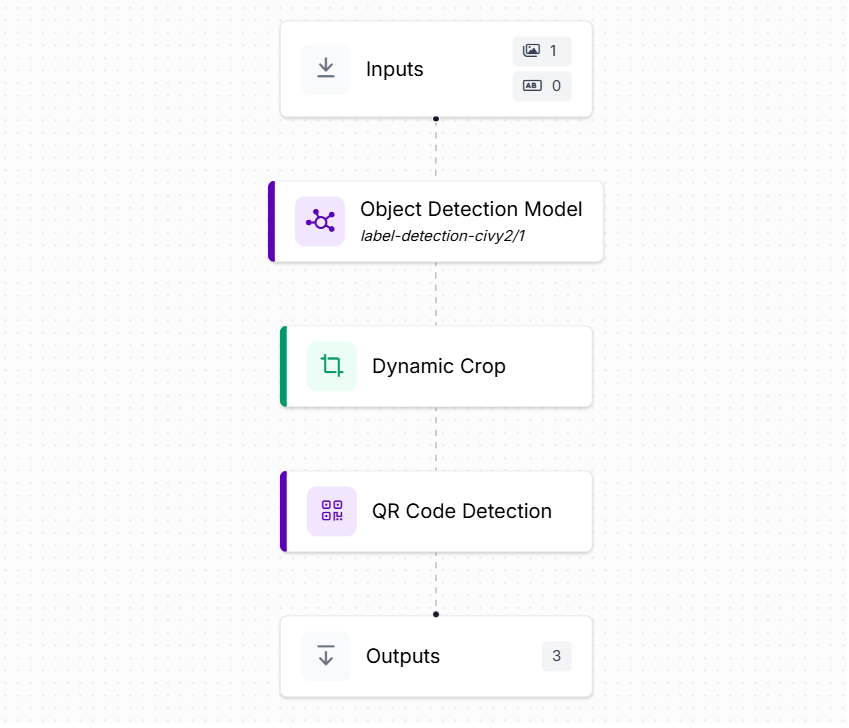
In the Object detection model block configuration specify that Class Filter property with class name to detect only the QR code only if your model detects multiple classes, as in our case.
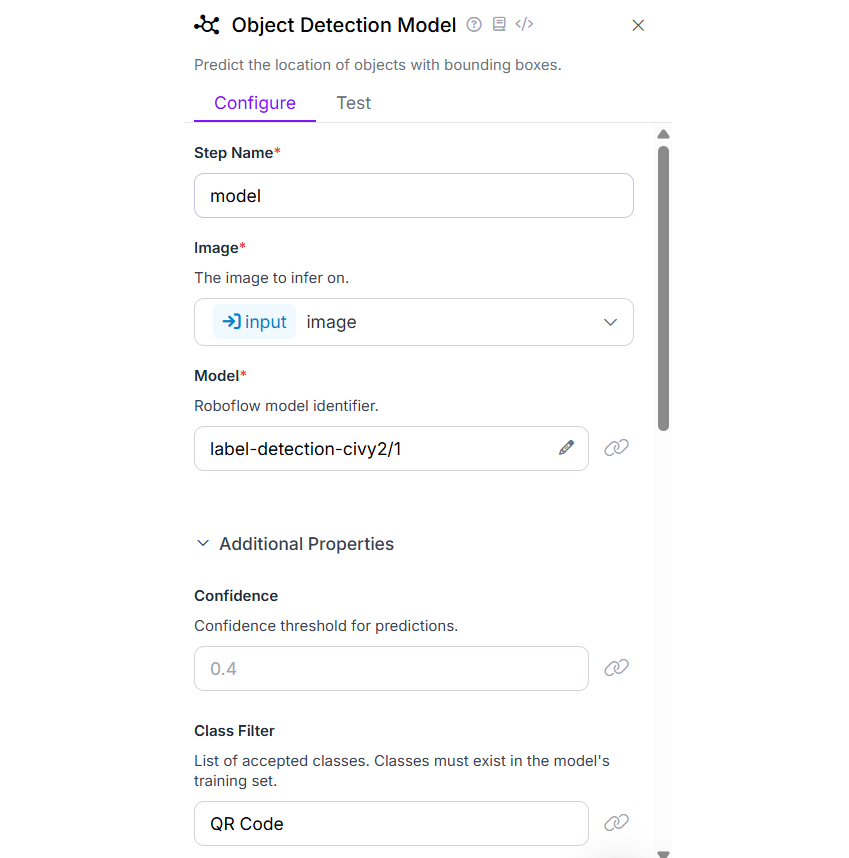
Running the workflow and uploading the image with QR code will generate output similar to following. It will return the QR code information as:
"data": "https://www.usps.com/"
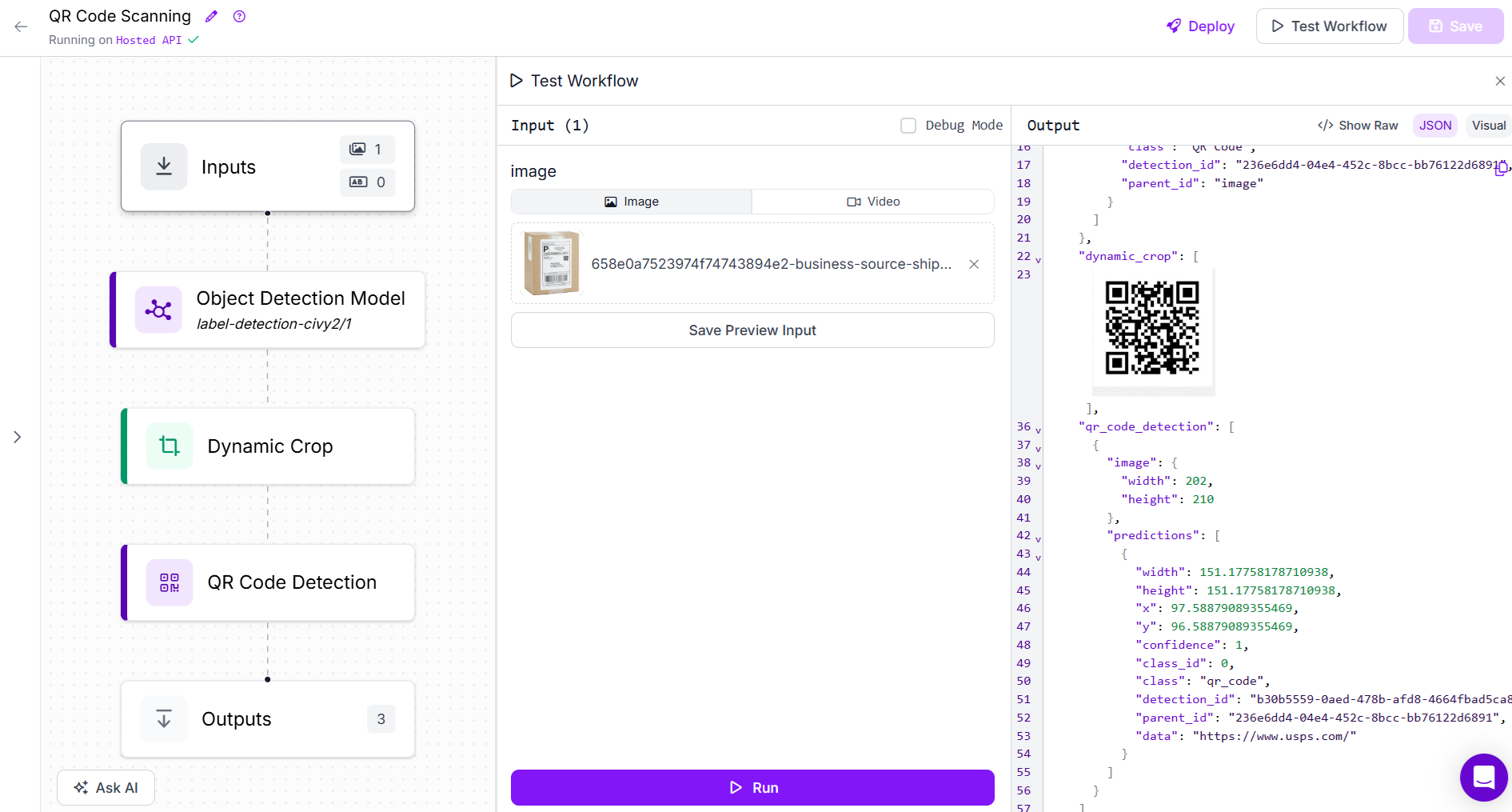
Step #2.3: Reading OCR
Documents or labels on pallets may contain any other information that need to be detected and processed. For example, list of items in the pallets. There are different techniques to extract OCR. Here we will make use of OCR model block in Roboflow workflow. The following is the workflow, almost similar to what we used in step 2.1 and 2.2 but with OCR Model block.
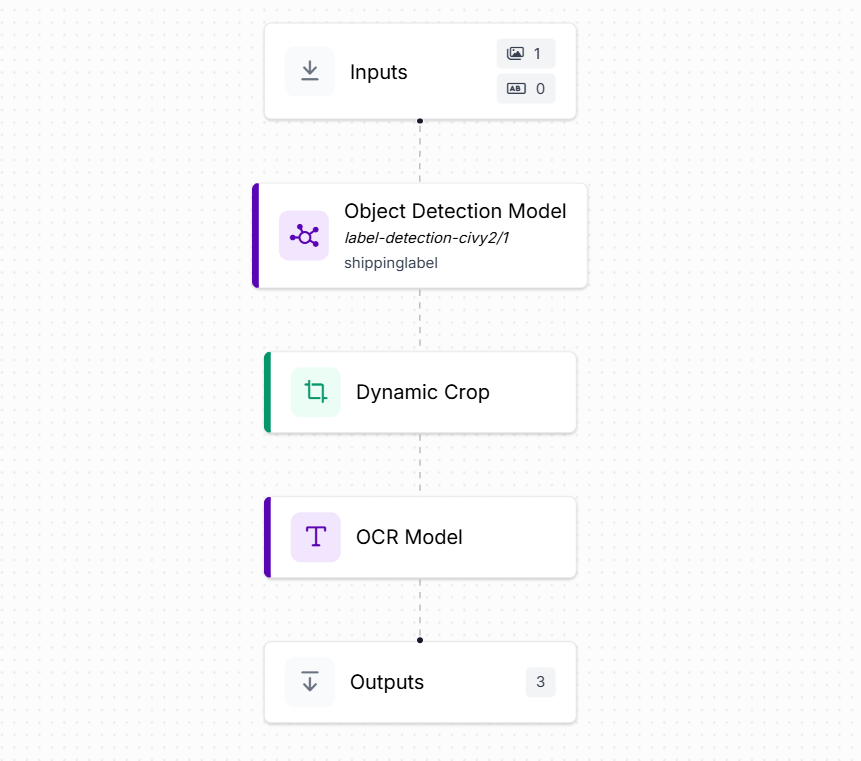
In the object detection model block configuration for this workflow, I selected the model built in Step 1 and set the Class Filter property to detect only the shipping-label class. This allows the model to extract all relevant data except the QR code and Barcode.
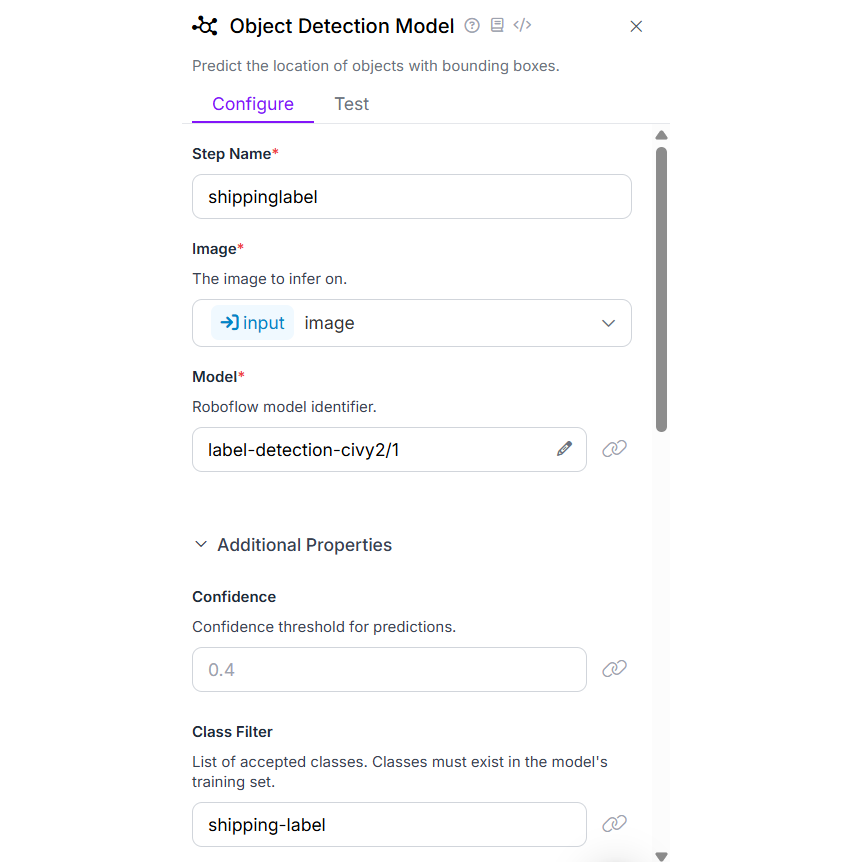
When you run the workflow and upload the image with product label, you will see output similar to the following. It will return all the text as shown in image below.
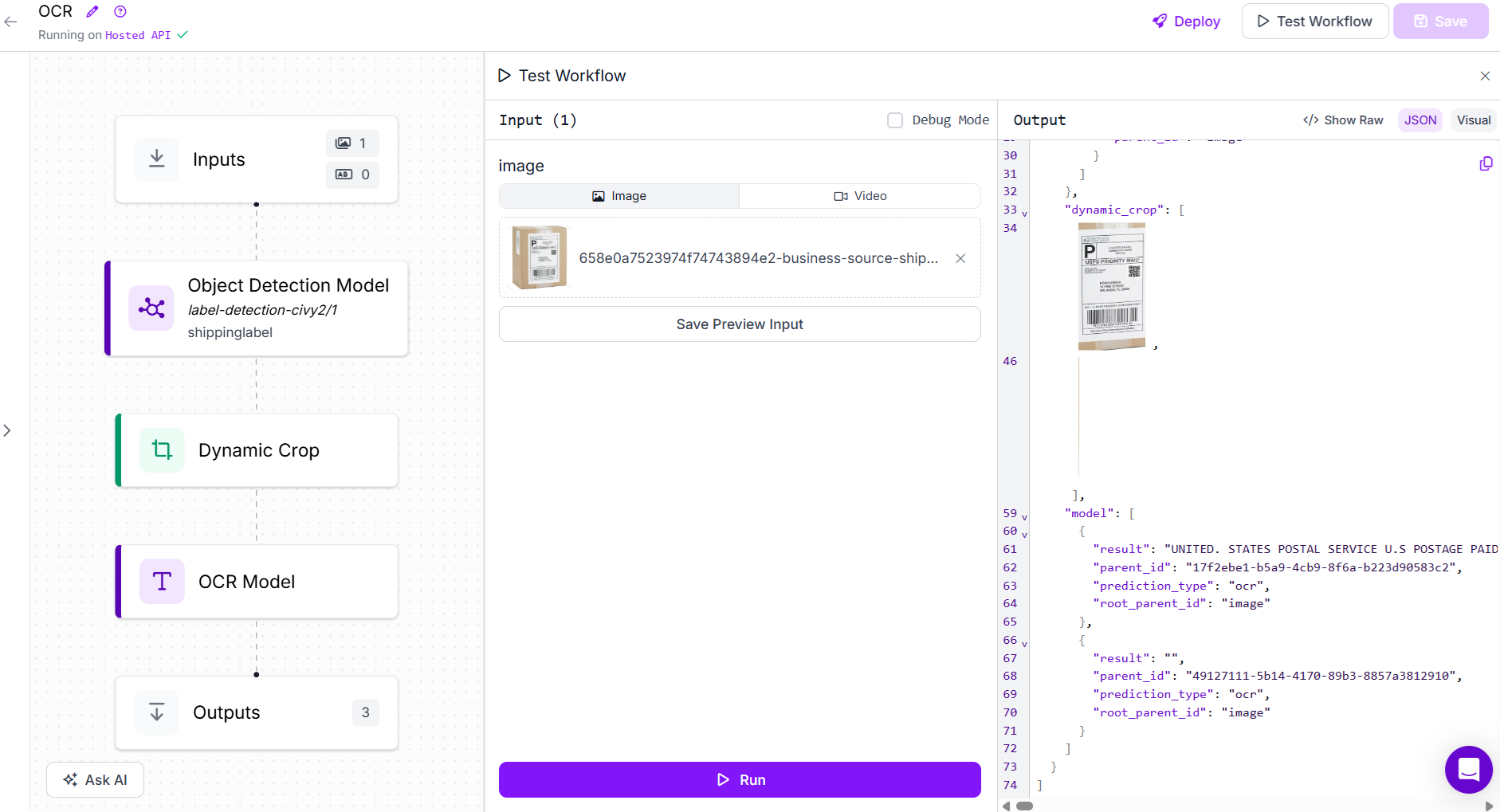
Step #3: Deploy the application
After building the Workflow application, it can be deployed in multiple ways. You can deploy it using Python, JavaScript, or as an HTTP/cURL request. Roboflow also provides the option to use the Workflow via its Hosted API or set up a local server. Additionally, Workflows can be deployed on edge devices for faster inference. There are various deployment options available. In this case, we use a Gradio app to create a GUI application that integrates the Barcode Reading workflow from Step 2.1. Simply copy and paste the following code to get started.
from inference_sdk import InferenceHTTPClient
import numpy as np
from PIL import Image
import gradio as gr
# Initialize the Inference client
client = InferenceHTTPClient(
api_url="https://detect.roboflow.com",
api_key="ROBOFLOW_API_KEY" # Replace with your actual API key
)
def extract_barcode_data(image: np.ndarray):
# Convert the numpy array to a PIL Image
pil_image = Image.fromarray(image)
# Run the workflow
result = client.run_workflow(
workspace_name="tim-4ijf0",
workflow_id="custom-workflow-9",
images={"image": pil_image},
use_cache=True
)
# Initialize a list to store barcode data
barcode_data_list = []
# Iterate through the results to extract barcode data
for item in result:
barcode_detection = item.get("barcode_detection", [])
for detection in barcode_detection:
predictions = detection.get("predictions", [])
for prediction in predictions:
barcode_data = prediction.get("data", "No data found")
barcode_data_list.append(barcode_data)
# Return the extracted barcode data
return "\n".join(barcode_data_list) if barcode_data_list else "No barcode data found."
# Define the Gradio interface
iface = gr.Interface(
fn=extract_barcode_data,
inputs=gr.Image(type="numpy"),
outputs=gr.Textbox(),
title="Barcode Data Extractor",
description="Upload an image to extract and display barcode data."
)
iface.launch()
The above code code sets up a web application that allows users to upload an image and extract barcode data from it. When a user uploads an image, it is sent to a Roboflow workflow for processing. The workflow analyzes the image and returns a response containing detected objects, including barcodes. The code then extracts barcode data from the response and stores it in a list. If barcode data is found, it is displayed as text; otherwise, a message indicating that no barcode data was found is shown. You will see the following output when you run the code.
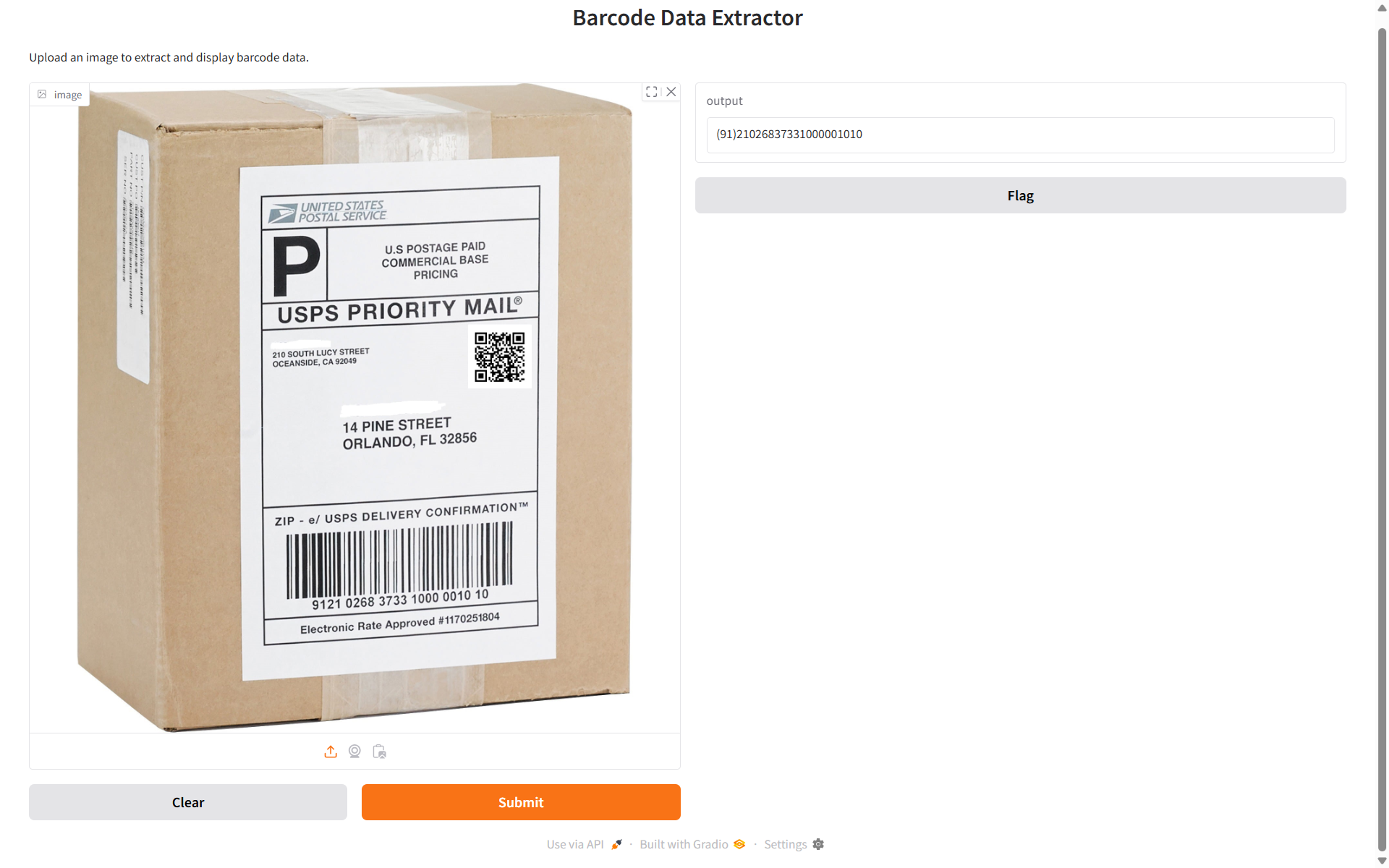
We have seen how effortless it is to build an application for industrial use cases like pallet scanning. Roboflow Workflow is a powerful tool that can be effectively utilized for such applications, enabling accurate and efficient barcode, QR code and OCR detection to streamline operations in warehouses, logistics, and inventory management.
Conclusion
Pallet scanning using computer vision is revolutionizing logistics, warehousing, and supply chain management by automating label detection, barcode and QR code recognition, and OCR-based text extraction.
In this blog, we explored the step-by-step process of building a pallet scanning application, from training a computer vision model to implementing workflows for barcode detection, QR code scanning, and OCR-based text recognition. Roboflow simplifies the development of pallet scanning applications by providing tools for dataset annotation, model training, and deployment.
Cite this Post
Use the following entry to cite this post in your research:
Timothy M.. (Mar 13, 2025). How to Scan Pallets using Computer Vision. Roboflow Blog: https://blog.roboflow.com/how-to-scan-pallets-using-computer-vision/