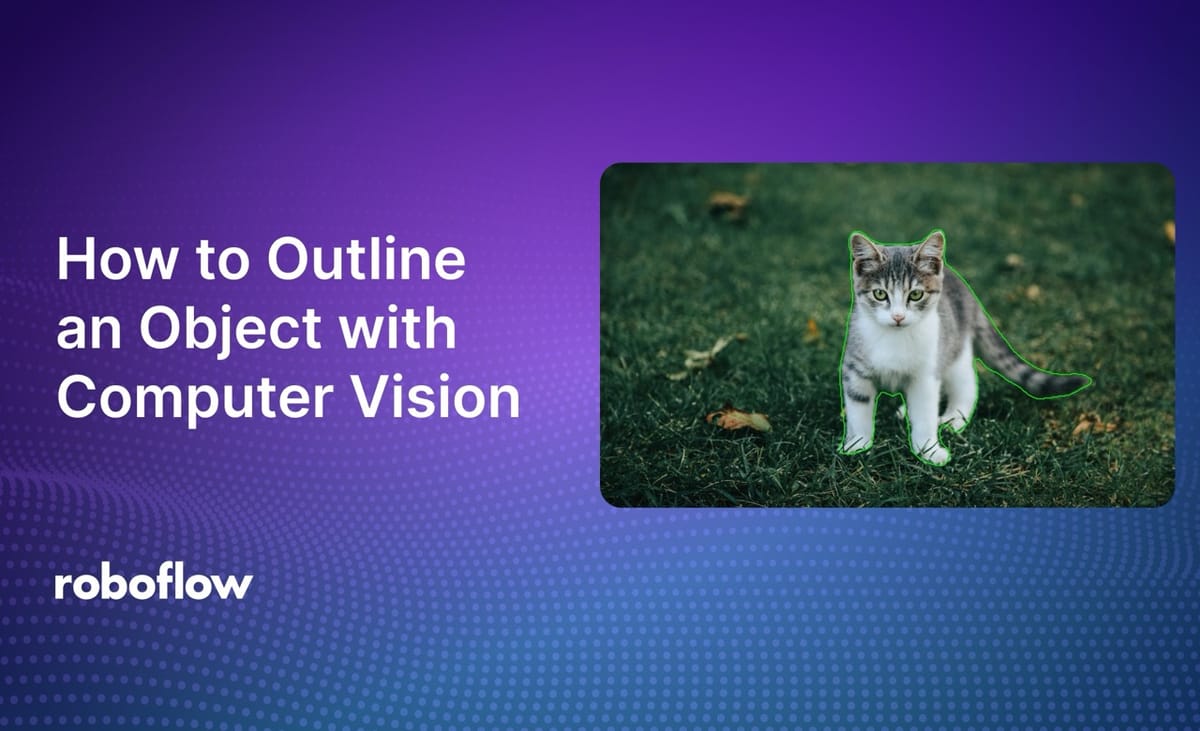
Calculating outlines for objects, also known as segmentation, is a technique in computer vision that precisely identifies the pixels that make up the boundary of an object.
Unlike just drawing a box around an object (as in object detection), outlining carefully traces the exact shape of the object, even if the object has a complex or irregular form. Outlining an object in an image or a video can be essential in many areas and form the basis of various applications.
Segmentation can help in many areas. For example, with regard to self driving cars, segmentation can help the vehicle accurately detect pedestrians, other cars, and obstacles. In medical imaging, doctors can use computer vision and segmentation to identify medical issues with greater accuracy and help with better diagnosis and treatment.
Segmentation and outlining objects also has applications in photo editing, where it can make tasks like removing backgrounds or isolating objects much easier.
In this article, we'll explore the concept of using computer vision to outline objects in an image and discuss the significance of segmentation in various applications. We'll also guide you through a coding example that showcases how to outline objects using popular tools like Roboflow and YOLOv8.
What is Object Outlining?
Object outlining allows you to calculate outlines around objects in an image. You can calculate object outlines using computer vision techniques. One approach is to use traditional computer vision approaches that search for boundaries in objects by color, although modern image segmentation models offer significantly better accuracy.
Instance segmentation can help you locate objects in an image and trace their exact shape, pixel by pixel. You can use instance segmentation to capture the precise outline of any object in an image. On the other hand, object detection focuses on finding and placing a bounding box around each object. It’s great for identifying and locating objects quickly, but it doesn’t show the object’s exact shape. Object detection is often used in applications like surveillance, where speed is more important than precision.
Many segmentation models are deployed in environments where speed matters – for example, in processing real-time video. As a result, fine-tuned models are often used, which can outline objects effectively and fast.
With that said, some foundation models, like Segment Anything 2, (SAM-2) offer high accuracy without fine-tuning. Foundation models like SAM-2, however, often take multiple seconds to run on an image and require a GPU.
How to Outline an Object Using Roboflow
Let’s calculate an object outline using Roboflow and YOLOv8 Segmentation, an image segmentation architecture.
To try using instance segmentation, you'll need to download an image to work with. We’ll be using this image of a cat.
The model we are using can segment 80 different objects, including common animals, vehicles, and more. You can also use any of the segmentation models available on Roboflow Universe with this tutorial, or models you have trained on or uploaded to Roboflow.
Step #1: Install Dependencies
To get started, install the inference library, which provides easy access to pre-trained models like YOLOv8. Roboflow Inference is a tool that can help you deploy these models directly into your applications for real-time segmentation.
You can install it using pip with the following command:
pip install inference
Step #2: Load the YOLOv8 Model
After installing the library, the next step is to load the pre-trained YOLOv8 model. Here's how to load the model:
import inference
# Load the YOLOv8n instance segmentation model
model = inference.get_model(model_id="yolov8n-seg-640")
Step #3: Run the Model
Once the model is loaded, the next step is to pass an image through it to generate object outlines. The process of analyzing an image using a model is known as running an inference on the image. The code snippet below will help you load the image you want to analyze and then use the model to predict the object outlines using instance segmentation.
import cv2
# Load an image
image = cv2.imread("path/to/image/file")
# Run the model on the image
results = model.infer(image)[0]
Here is the input image we are using.
Step #4: Visualize the Output
After running the inference, the next step is to visualize the segmented objects by drawing the outlines on the original image. It helps you see how accurately the model has segmented the pixels of each object. The code below extracts the points that outline each object’s pixels and draws them in green on the image. The final result provides a clear view of how the model segmented each object in the scene.
import numpy as np
# Create a copy of the image to draw on
output_image = image.copy()
# Loop through predictions and draw the contours
for prediction in results.predictions:
points = np.array([(p.x, p.y) for p in prediction.points], dtype=np.int32)
cv2.polylines(output_image, [points], isClosed=True, color=(0, 255, 0), thickness=8)
# Save the image with the drawn contours
cv2.imwrite('result.jpg', output_image)
Here is the output with the object in the image outlined.
Challenges and Considerations
Outlining objects accurately in a complex image using computer vision isn’t always simple. One of the biggest challenges is handling overlapping objects. When objects overlap or partially block each other (a situation known as occlusion), even advanced models like YOLOv8 can struggle to separate and define their boundaries.
Complex shapes add another layer of difficulty. Many models are trained using simple, regular shapes, so when they encounter objects with intricate or irregular outlines, capturing every detail accurately can be a real challenge. Segmentation becomes even tougher when you throw in factors like varying light conditions, changes in the object’s orientation, or when parts of the object are hidden. All these factors can affect how well a model can trace the object’s edges.
Aside from challenges posed by the image itself, there’s always a need to balance accuracy and speed. Models like YOLOv8 prioritize speed but might sacrifice some accuracy in tracing object outlines. More accurate segmentation models, on the other hand, typically require more computational power and are slower, which can be a drawback for tasks needing quick processing.
Aiming to achieve this balance also affects hardware requirements. For example, training complex models like Mask R-CNN demands powerful GPUs due to the large number of parameters involved in learning to create pixel-level masks. In real-time applications, specialized hardware like TPUs or AI accelerators can help improve performance and reduce delays. However, if you’re working with devices that have limited resources, like edge devices, you’ll need to optimize the models to ensure they run efficiently without straining the hardware.
Object Outlining Applications and Use Cases
Now that we've understood how to outline an object in an image using computer vision and the challenges that may be involved, let's take a more in-depth look at how this can be applied to different situations.
Autonomous Vehicles
Instance segmentation makes it possible for self-driving cars to detect and classify everything around them with incredible precision by creating pixel-level masks that outline the exact shape of each object. Whether it’s a pedestrian, another car, or a traffic sign, this detailed understanding helps the vehicle navigate complex situations like crowded city streets or construction zones.
A great case study of outlining objects using computer vision in autonomous vehicles is NVIDIA’s DriveAV system that uses panoptic segmentation. Panoptic segmentation combines semantic segmentation (classifying every pixel in an image into categories like 'road' or 'car') with instance segmentation (which also tells apart between different instances of the same object, like multiple cars on the road).
Tesla also relies on advanced computer vision in its Autopilot and Full Self-Driving (FSD) systems. These systems use instance segmentation to get a detailed view of the vehicle’s surroundings, helping it make smart decisions on the road. For example, they help the car decide when to stop, speed up, or change lanes.
Medical Imaging
Instance segmentation can play a key role in medical imaging and support tasks like diagnosing and treating various health conditions. By accurately outlining and identifying objects like organs, tumors, and tissues within an image, this technique gives doctors a detailed view that’s essential for making correct diagnoses, planning treatments, and keeping track of diseases.
For example, in MRI scans, instance segmentation can distinguish between a tumor and healthy brain tissue. Doctors can closely examine and diagnose conditions like gliomas or meningiomas. Instance segmentation can also be used in other medical procedures, such as endoscopy and radiography. It speeds up and improves the accuracy of identifying abnormal areas. With insights gained from computer vision techniques, doctors can develop more targeted and personalized treatment plans.
Image Editing and Augmentation
Instance segmentation can also be a reliable tool for image editing and augmentation. It can make complex editing tasks much easier and more intuitive. For example, you can use instance segmentation to precisely select and manipulate individual objects within an image. If you have a photo of a crowded street and want to change the color of a specific car or replace a building in the background, instance segmentation makes it easier for you to isolate that car or building.
Warehouse Inventory Management
Managing warehouses is vital for businesses that depend on smooth operations to keep their supply chains running seamlessly. Even a small hiccup in warehouse management can cause big disruptions that affect everything from productivity to customer satisfaction. That’s why it’s so important to make sure that warehouse operations are well-organized and efficient.
Instance segmentation can modernize how warehouses manage their inventory. Warehouses can use technologies like LiDAR and high-definition cameras to capture detailed images of storage spaces. When combined with instance segmentation and object tracking, these technologies can precisely identify and manage inventory, even in complex environments. The system can integrate with robots to automate sorting and transportation tasks, and increase safety and accuracy within the warehouse.
Conclusion
Accurately outlining objects in images and videos is essential in various computer vision applications. By capturing the exact shape and boundaries of objects, instance segmentation can enhance the performance and reliability of different innovations.
For anyone interested in computer vision projects, experimenting with models like YOLOv8 can be a great opportunity to try using instance segmentation. Whether you're working on real-time applications or detailed image analysis, these models can improve the accuracy and effectiveness of your projects and open up new possibilities for innovation.
Keep Exploring
Check out these resources to learn more:
Cite this Post
Use the following entry to cite this post in your research:
Contributing Writer. (Sep 3, 2024). How to Outline an Object with Computer Vision. Roboflow Blog: https://blog.roboflow.com/outline-objects-computer-vision/