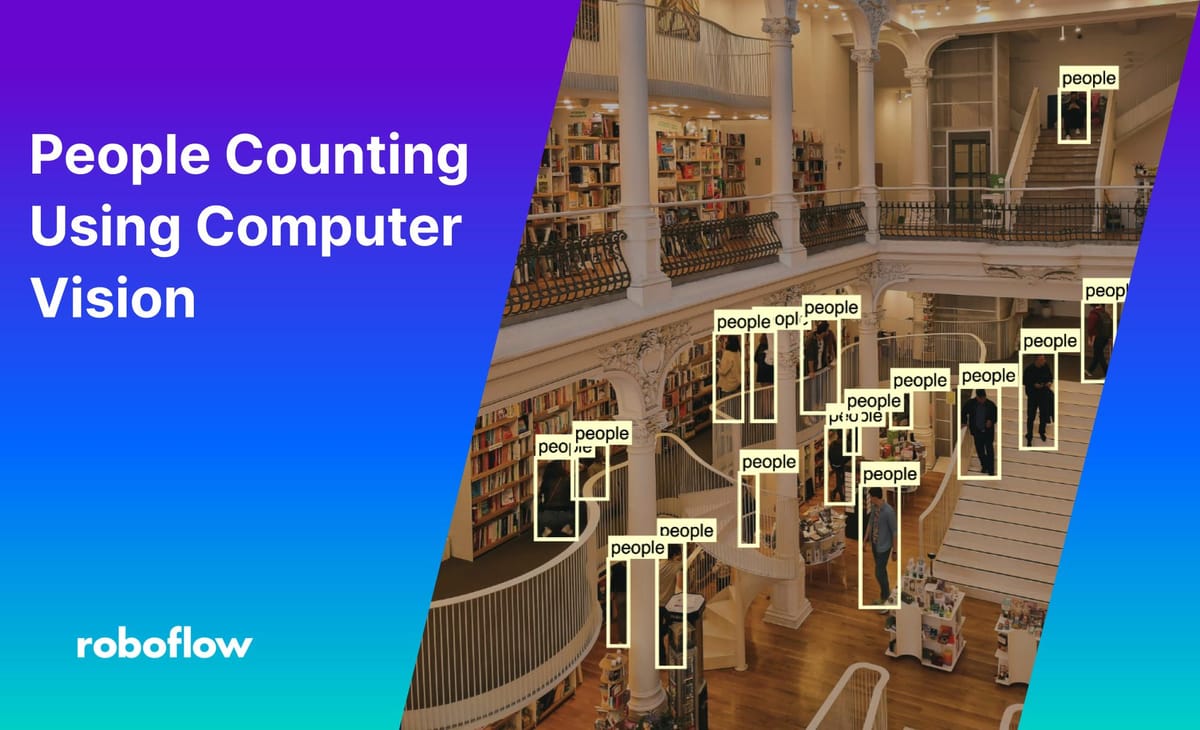
Introduction
Counting and keeping track of a large number of people entering and exiting an event can be challenging, especially when security is a priority. Traditional methods of monitoring people make it difficult for security officials to keep track of everyone in real-time. However, advancements in AI technologies like computer vision can step in as an innovative solution here.
Counting people using computer vision is a great way to analyze how many people are in a particular area. People counting is useful for many situations, like managing an entry and exit count at events, designing better store layouts for retail businesses, and boosting security in public places. By knowing the exact number of people in a location at a given time, businesses and organizations can make better decisions. For example, they can adjust the number of staff needed, improve customer service, and ensure safety rules are followed.
In this article, using a people detection model from Roboflow, we’ll create a dependable and effective solution for counting people that can be used in various real-life situations. Let's dive into the details.
Applications of People Counting
Before we take a look at the code for this project, let’s first explore some of the potential applications of using computer vision based people counting solutions.
Smart Cities
Cities can become much smarter, safer, and easier to navigate by counting and keeping track of how many people use different areas within the city. City planners can use this information to decide what and where to build next.
For instance, knowing where people walk can help them create better walking paths and bus routes. Similarly, understanding the popularity of public parks allows them to improve these spaces for everyone.
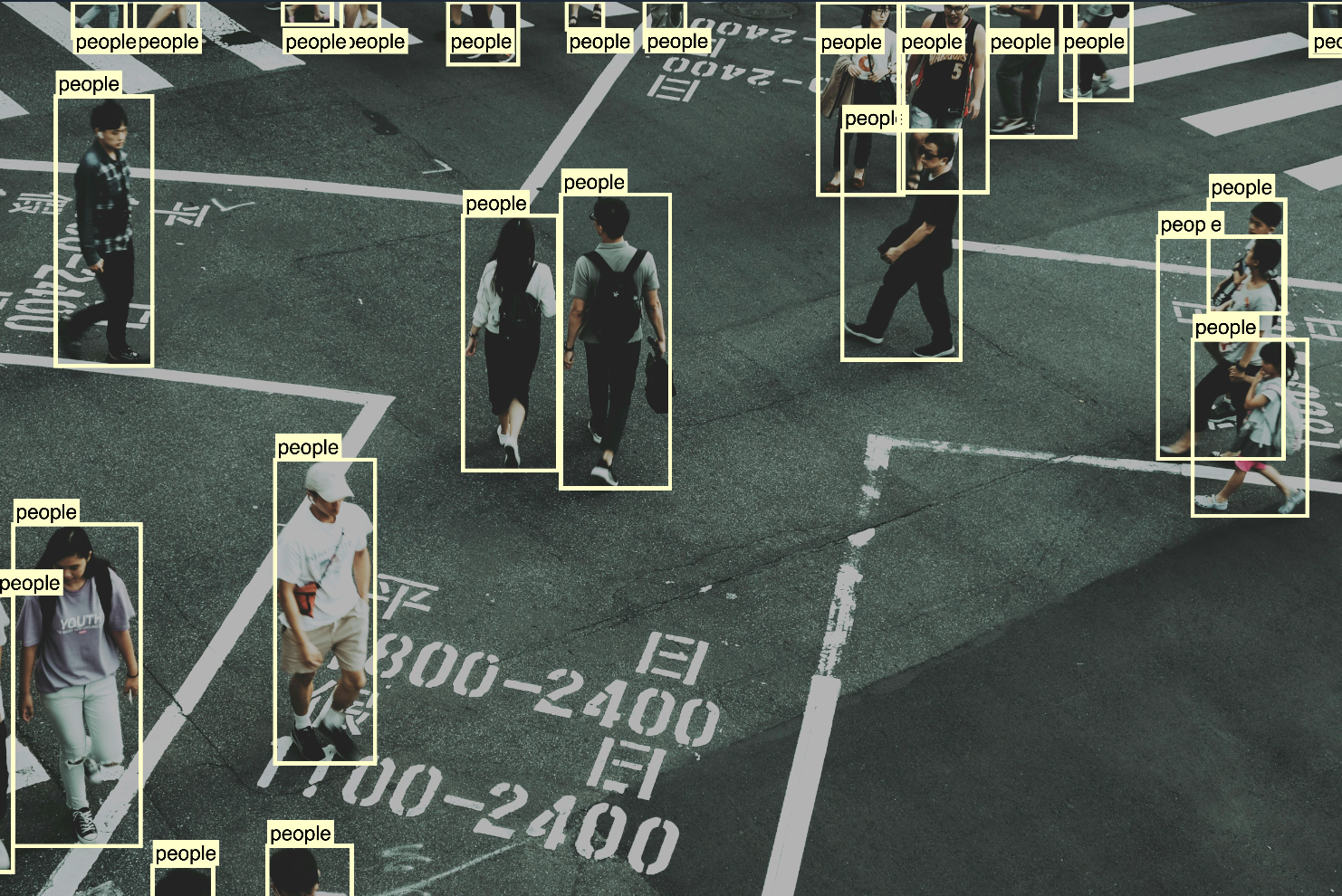
This technology also helps keep different areas safe. By counting how many pedestrians are on the roads, cities can adjust traffic lights, add more crossing lines, and improve public transportation and reduce traffic jams. It's also important to monitor how many people are at events, and people counting helps avoid overcrowding-related issues.
Retail
By tracking how many customers enter the store, how long they browse, and how many make purchases, retailers can gain valuable insights into customer behavior. This information can then be used to improve store operations and boost sales. Retailers can optimize the layout of their stores to improve traffic flow and product visibility by seeing how customers move around the store.
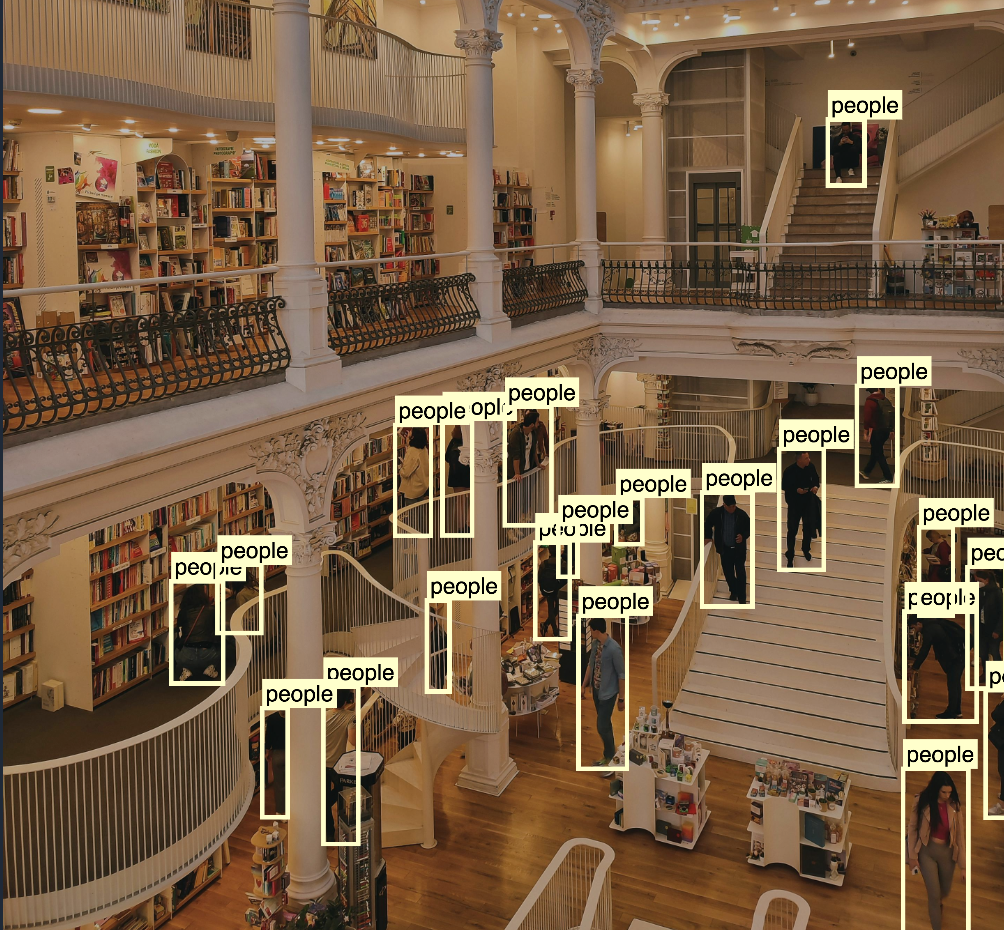
Also, knowing peak customer hours allows for better staffing decisions. It can directly result in shorter lines, happier customers, and less stressed employees.
Transportation
Computer vision-based people-counting solutions can help track how many people are using buses, trains, and other services at any given time. Real-time data allows transportation agencies to adjust their services on the go. During rush hour, they can add more vehicles to prevent overcrowding. When the rush is slowing down, they can reduce service to save money and resources.
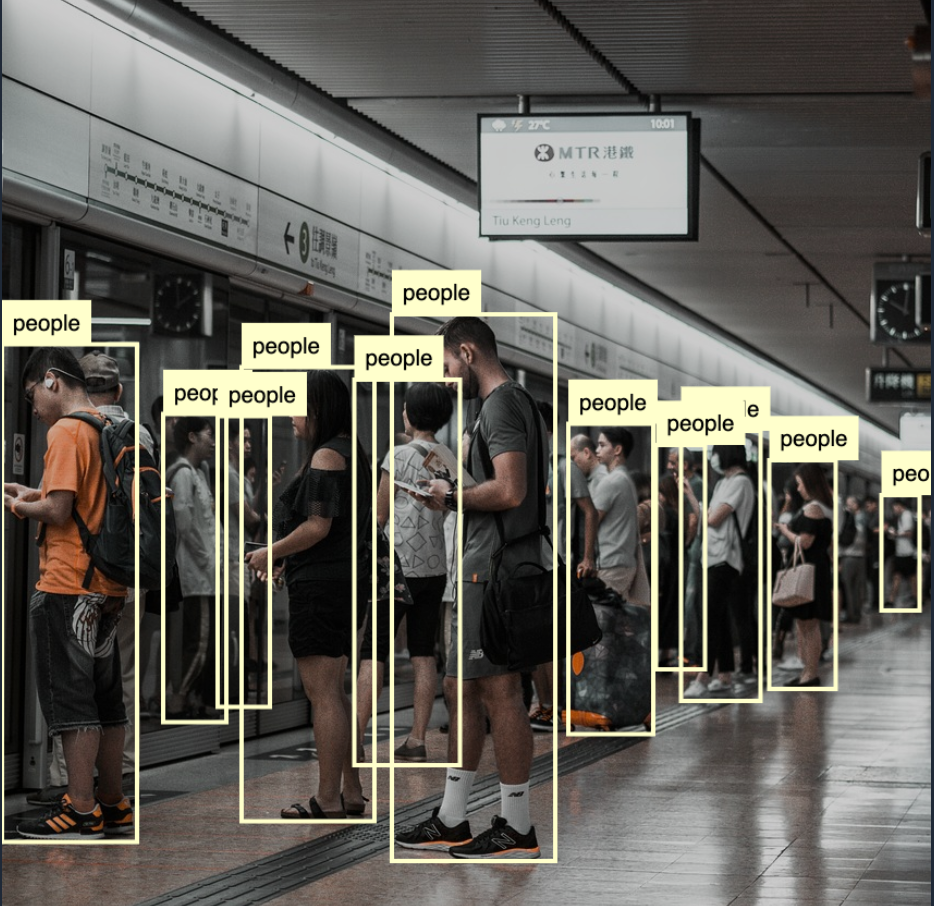
It also helps them respond to unexpected events like bad weather or accidents by rerouting or adding extra services. Plus, by analyzing long-term data, transportation companies can see usage trends and plan for the future, making public transportation a more attractive option for everyone.
Marketing and Advertising
People counting using computer vision has many applications across marketing and advertising. Using this tech, businesses get a clear idea of the amount of people visiting their stores. The real time foot traffic data collected by such tech can help improve marketing campaigns by placing ads, promotions, and premium products in busy areas.
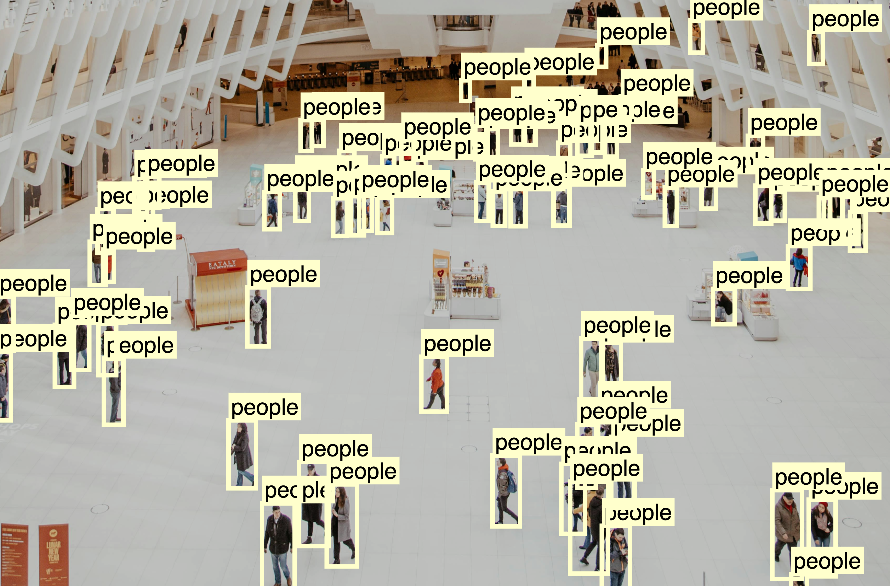
Marketers can also use the data for targeted advertising, after analyzing the demography and behavior of the customers, personalized ads can be created.
Crowd Management
If you've ever organized an event then you'd know just how difficult it is to monitor the crowd that attendees the event. People-counting technology provides real-time crowd data to keep everyone safe by preventing overcrowding and making sure capacity regulations are being followed.
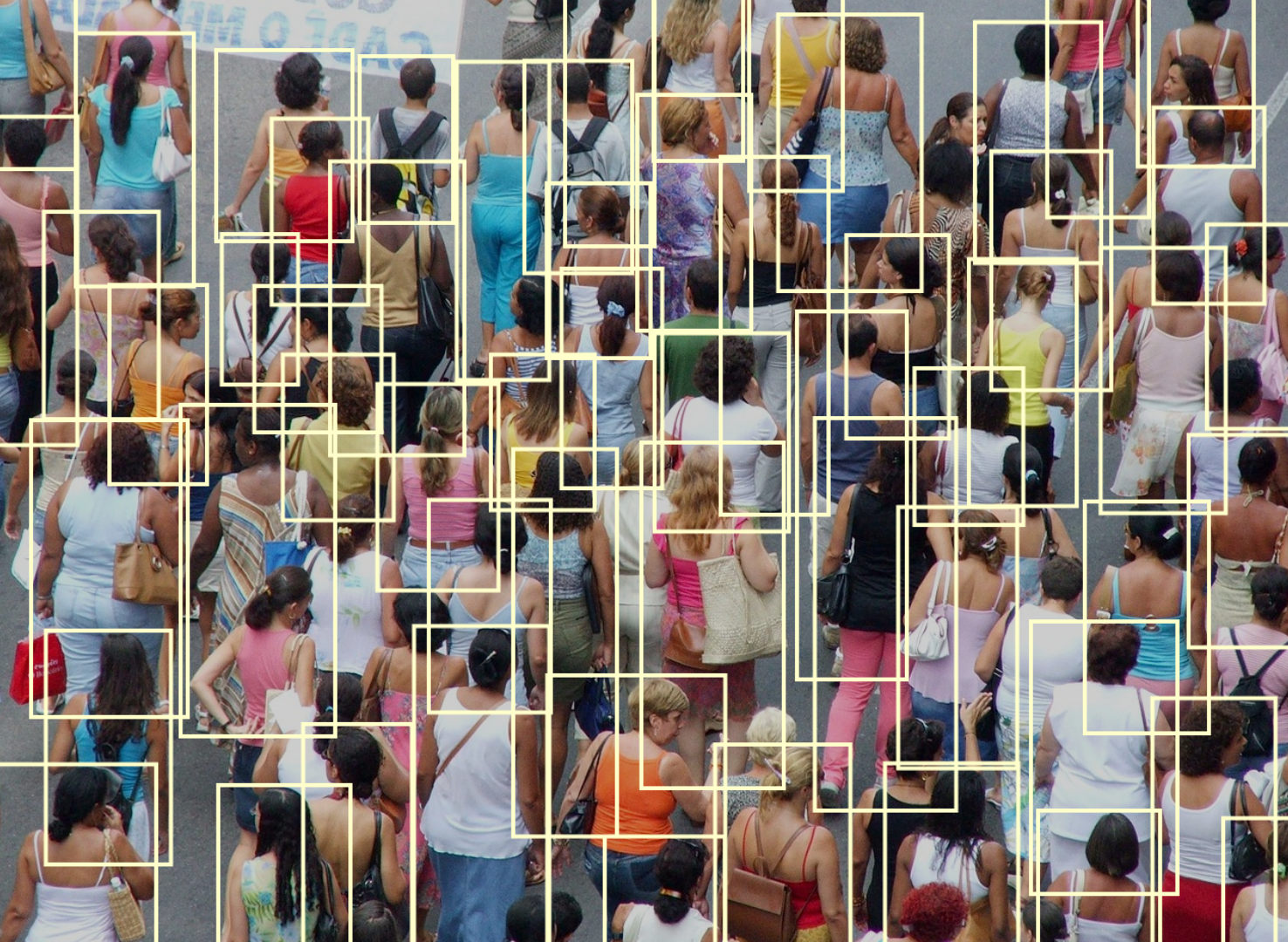
That's not all! The data also helps organizers make the most of their resources, placing staff and amenities where attendees need them most. This means less waiting in lines and smoother traffic flow throughout the event.
People Counting with Computer Vision
Now that we’ve explored some of the many applications of computer vision-based people counting, let’s take a look at how to implement such a solution. We’ll walk through a people counting project using a custom-trained model. We will cover setting up the environment, loading the custom model, and implementing the counting logic with a code example.
Step 1: Setting Up the Environment
To begin, we need to set up our development environment. The first step is to install the necessary tools and libraries required for our project. Open a terminal or command prompt and run the following command to install the required libraries:
!pip install opencv-python matplotlib inference_sdk
Step 2: Loading the Custom Trained Model
Next, we will load the custom-trained model from Roboflow. The model we’ll be using is trained to detect people in various scenarios using a generalized dataset. The training data for this model comes from various projects to be able to perform well across different contexts, annotation sizes, and camera types.
To access the model, visit the model's page at Roboflow Universe as shown below. Roboflow Universe serves as a central hub for open-source computer vision datasets and models, offering an extensive collection of over 200,000 datasets and 50,000 pre-trained models. We’ll be accessing the model through the provided API for inference.
Upon scrolling down, you’ll see a piece of sample code that shows how to infer on local and hosted images. Be sure to note the model ID and version number from the sample code. In this case, the model ID is “people-detection-o4rdr,” and it’s the seventh version of the model. This information will come in handy when we assemble our inference script.
Step 3: Implementing the People Counting Logic
Now, we will write the code to load the custom-trained model, perform inference on images or video streams, and display the results with bounding boxes and count overlays. But first, we need to initialize the InferenceHTTPClient with the API URL and your API key to load the custom-trained model.
from inference_sdk import InferenceHTTPClient
import cv2
import matplotlib.pyplot as plt
# Initialize the InferenceHTTPClient
CLIENT = InferenceHTTPClient(
api_url="https://detect.roboflow.com",
api_key="API_KEY"
)
Step 4: Perform Inference on Images or Video Streams
Next, we can use the infer method of the InferenceHTTPClient to perform inference on an image. This method runs the image to the model and returns the predictions.
# Perform inference on the image
result = CLIENT.infer("your_img.jpg", model_id="people-detection-o4rdr/7")
Step 5: Count People in the Images or Videos
The number of people detected is the length of the prediction list returned by the infer method.
# Count the number of predictions (people detected)
num_predictions = len(result['predictions'])
Step 6: Display Results with Bounding Boxes and Count Overlays
Finally, we can draw bounding boxes around the detected people and display the count of people in the image. We will use OpenCV for drawing and Matplotlib for displaying the image.
# Load the image using OpenCV
image = cv2.imread("b.jpg")
height, width, _ = image.shape
# Extract prediction details and draw bounding boxes
for prediction in result['predictions']:
x = int(prediction['x'] - prediction['width'] / 2)
y = int(prediction['y'] - prediction['height'] / 2)
w = int(prediction['width'])
h = int(prediction['height'])
# Draw the bounding box on the image
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
label = f"{prediction['class']}:{prediction['confidence']:.2f}"
cv2.putText(image, label, (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# Convert the image from BGR to RGB (OpenCV uses BGR by default)
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Set the size of the output image
plt.figure(figsize=(12, 8))
# Display the image using matplotlib
plt.imshow(image)
plt.axis('off') # Hide the axis
plt.title(f"Number of predictions: {num_predictions}")
plt.show()
An example input image is shown below.
Here is the output of analyzing the example input image.
Challenges and Considerations for People Counting Software
Implementing people counting technology comes with several challenges and considerations that need to be made to make sure it works well and follows ethical guidelines. Here are some points to keep in mind:
- Anonymizing Data and Protecting Privacy: Techniques like blurring people's faces can anonymize data. Processing video on devices (edge computing) adds another layer of privacy protection.
- Handling Occlusions in Crowded Scenes: Advanced algorithms can help us detect people even when they're close together. Using multiple cameras from different angles gives a complete picture of the crowd.
- Ensuring Real-Time Performance: Video processing can be optimized to reduce delays. Using special AI hardware can make the analysis faster too. The best method depends on what the application needs.
- Scaling to Large Deployments: Larger tasks can be broken down into smaller parts for efficient processing. On-device processing can also allow the system to scale while keeping data private. Cloud platforms can handle massive amounts of data for analysis.
Future Directions in People Counting
Computer vision-based solutions for counting people are getting better and will likely be used more often in smart cities in the future. Integrating it with sensors like thermal cameras that can detect body heat which makes it easier to count people in all conditions. The combination of different sensors makes the overall system more accurate and reliable.
The data gathered from the system can help smart cities manage traffic, public safety, and resource allocation. It can also be used to predict future trends, like busy times or areas, allowing for better planning and decision-making. People counting using computer vision can help cities and businesses work more efficiently and safely.
Conclusion
People counting applications, powered by high-definition cameras and computer vision algorithms, can be a game changer for businesses and organizers. It tracks how many people are in a store, at an event, or even on public transport, all in real time. This gives them the power to make smarter choices, like optimizing staff levels, improving layouts, and ensuring everyone's safety. As this tech gets even better, we can expect it to be used everywhere, from smart cities to your local park, making sure our lives are safer and more convenient without us even realizing it!
Keep Reading
- Level Up Your Ecommerce Business with Computer Vision.
- Learn How to Monitor and Analyze Retail Queues.
Cite this Post
Use the following entry to cite this post in your research:
Contributing Writer. (Jul 19, 2024). People Counting Using Computer Vision. Roboflow Blog: https://blog.roboflow.com/people-counting-computer-vision-software/