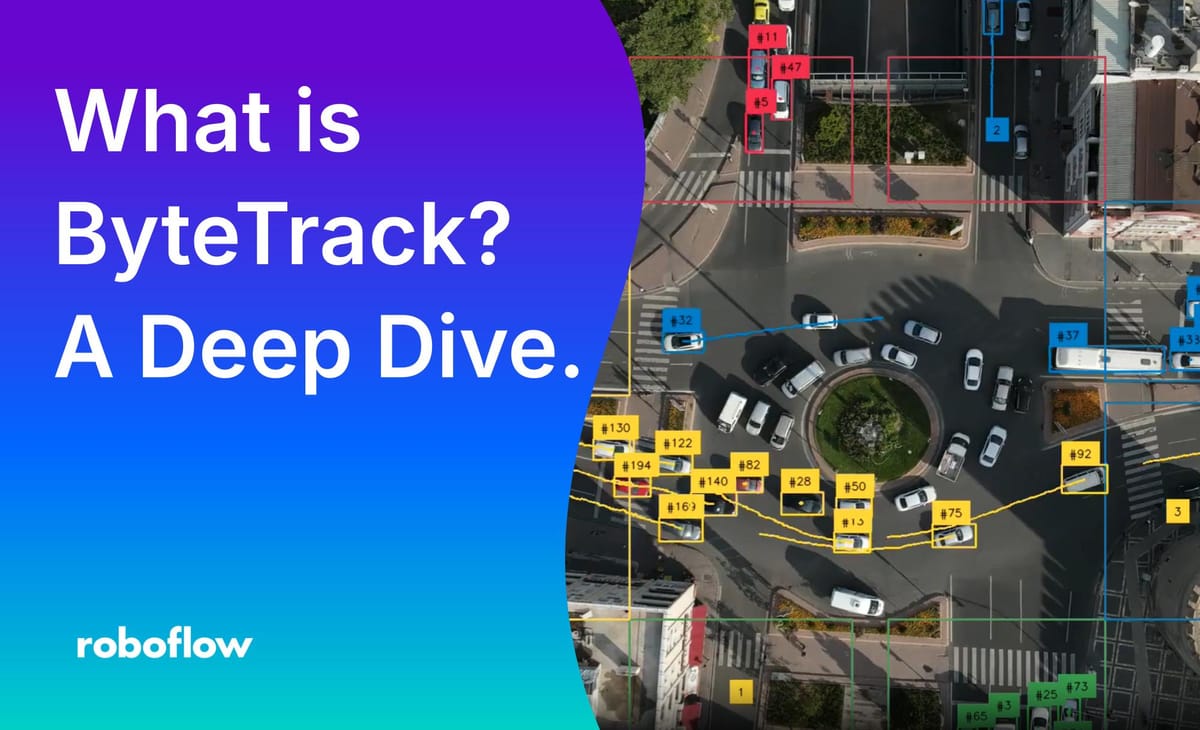
Introduction
Detecting and tracking multiple objects in a video in real-time can be tricky. Luckily, the computer vision community has developed object-tracking algorithms to tackle this task over the years. These algorithms aim to identify and follow objects as they move through a video.
A great example of these algorithms is ByteTrack. It can detect and continuously track multiple objects by giving each one a unique ID. Unlike other algorithms, ByteTrack considers all detected objects (not just high-confidence ones). By doing so, it can improve its tracking accuracy even in challenging conditions like occlusion.
In this article, we'll dive deep into ByteTrack and see how it works and why it's such a valuable tool for applications like autonomous driving, sports analysis, and manufacturing. Let’s get started!
How ByteTrack Works
ByteTrack begins by detecting objects in video frames using an object detection model. The model draws bounding boxes around each detected object, and these bounding boxes come with confidence scores that indicate how certain the model is about each detection.
After detecting objects, the heart of ByteTrack - its data association module - connects these detection boxes with tracklets. A tracklet is essentially a short sequence of frames where an object has been consistently detected and tracked. By associating detection boxes with tracklets, ByteTrack makes sure that objects are accurately tracked over time, even as they move through the video. The algorithm also uses a gating mechanism to filter out redundant detections.
The data association process consists of the following two stages:
- Stage 1: ByteTrack starts by matching high-confidence detection boxes (those with scores above a certain threshold) with tracklets. It helps ensure that the most reliable detections are correctly paired with the right tracklets and reduces the chances of mixing up object identities.
- Stage 2: ByteTrack then matches the remaining low-confidence detection boxes with tracklets by comparing how similar they are. It measures similarity using two methods: how much the boxes overlap (called intersection over union, or IoU) and how similar the objects look (using cosine similarity of appearance features). Stage 2 helps catch any missed matches.
These two stages of the data association process make the ByteTrack algorithm highly effective. ByteTrack benchmarks are quite high when it comes to tracking metrics like MOTA (Multiple Object Tracking Accuracy) and IDF1 (Identification F1 score). MOTA measures the overall tracking accuracy, and IDF1 evaluates the ability to correctly identify objects across frames. Compared to other methods, ByteTrack has higher MOTA and IDF1 scores.
Use Cases for ByteTrack
Now that we’ve understood what ByteTrack is and the basics of how it works, let’s explore some applications where it can really shine.
Tracking in Sports Analytics
Algorithms like ByteTrack can extract valuable insights from video playback footage. Coaches can use insights from this analysis to measure various players’ performance metrics. The data obtained can help the players identify their strengths and weaknesses and also help the coaches/managers devise better training strategies.
For example, in basketball games, the ByteTrack algorithm can be used to track the players' positions, movements, and interactions. The tracking data can be used to analyze offensive and defensive plays. These systems make the overall game-viewing experience for fans more entertaining and insightful by making it possible to create automated highlight reels and player statistics.
Tracking in Autonomous Vehicles
Multiple object tracking is one of the most essential computer vision features of self-driving cars. These vehicles require an accurate perception of the environment around them to work properly. The ByteTrack algorithm can detect and track the position and movement of one or more objects around these cars. It is crucial for tasks like collision avoidance, path planning, etc.
By maintaining consistent object identities, even when objects are partially occluded or moving rapidly, ByteTrack can help autonomous vehicles anticipate potential hazards and react accordingly. It can also be used to estimate object trajectories so that autonomous vehicles can predict the behavior of other road users and plan safe maneuvers. Popular autonomous car companies like Tesla and Waymo use such AI technologies for autonomous driving.
Tracking in Manufacturing
Another great application of multiple object tracking algorithms is tracking products on assembly lines. ByteTrack can be used in manufacturing to track products moving on a conveyor belt. When products are given unique IDs and tracked, manufacturers can monitor each product's movement and progress through all the different stages of production. They can also optimize the efficiency of production, identify any bottlenecks, and improve overall quality control.
For instance, ByteTrack can be used to detect and ID products with defects or errors. Since these defective products are being tracked with unique IDs, they can be easily singled out and removed from the production line.
How to Use ByteTrack
Now that we've explored some of the use cases of ByteTrack, let's dive into a step-by-step coding example to see how you can use it in your projects. ByteTrack can be easily added to any Python-based workflow that uses popular object detection models like YOLOv8. In this example, we'll use ByteTrack to track people in a video.
Step 1: Setting Up the Environment
To begin, we need to set up our development environment. The first step is to install the necessary tools and libraries required for our project. Open a terminal or command prompt and run the following command to install the required libraries:
pip install supervision inference
After installing these packages, you have all the dependencies needed to perform inference using the Roboflow platform.
Step 2: Downloading a Video to Analyze
Supervision, an open-source Python package with a range of utilities, offers you the ability to download a video of people walking. You can run the following code to download the video.
from supervision.assets import download_assets, VideoAssets
download_assets(VideoAssets.PEOPLE_WALKING)
Here is a glimpse of the input video for your reference.
Step 3: Detecting and Tracking People Using YOLOv8 and ByteTrack
Now that we have our environment set up and the video ready, let's move on to detecting and tracking people in the video using YOLOv8 and ByteTrack.
In this step, we'll begin by importing the necessary libraries for handling arrays, model inference, and video processing. We’ll then load the YOLOv8 model, which is a great option for detecting and localizing objects in videos. Be sure to use your API key to load the YOLOv8 model. After that, we’ll set up ByteTrack. We’ll also prepare tools to draw boxes and labels around the detected objects.
Next, we’ll create a callback function. This function will process each frame of the video by first detecting objects using YOLOv8 and then updating the tracking information with ByteTrack to ensure each object is consistently identified across frames. It will also add annotations like bounding boxes and labels to the frame. Finally, we’ll pass this callback function to the video processing utility, which will apply it to every frame of the video, resulting in a fully annotated and tracked video as the output.
import numpy as np
import supervision as sv
from inference.models.utils import get_roboflow_model
model = get_roboflow_model(model_id="yolov8n-640", api_key="Your_API_KEY")
tracker = sv.ByteTrack()
box_annotator = sv.BoxAnnotator()
label_annotator = sv.LabelAnnotator()
def callback(frame: np.ndarray, _: int) -> np.ndarray:
results = model.infer(frame)[0]
detections = sv.Detections.from_inference(results)
detections = tracker.update_with_detections(detections)
# Create labels with class names and tracker IDs
labels = [
f"#{tracker_id} {pred.class_name}"
for pred, tracker_id
in zip(results.predictions, detections.tracker_id)
]
annotated_frame = box_annotator.annotate(
frame.copy(), detections=detections)
return label_annotator.annotate(
annotated_frame, detections=detections, labels=labels)
sv.process_video(
source_path="people-walking.mp4",
target_path="result.mp4",
callback=callback
)
When the code is executed successfully, you’ll get an output file, ‘result.mp4’, with multiple objects being tracked by ByteTrack, as shown below.
Challenges and Considerations
It’s true that ByteTrack offers many benefits and advantages and can be used across various industries, but it’s good to be aware of the challenges involved as well. ByteTrack can struggle to track objects when something blocks the objects from view or when objects change how they look or move quickly out of frame.
Tracking small objects is also a unique challenge for the ByteTrack algorithm. These tasks are difficult because small objects have limited pixel representation and can easily be hidden behind larger objects. It often results in inaccurate bounding boxes and difficulties in maintaining consistent object identities.
Another challenge concerns real-time performance. Such algorithms require a lot of computational resources, which can be an issue for devices with limited resources like embedded systems. It is important to find the right balance between how well the algorithm works and how fast it runs.
Conclusion
ByteTrack is a computer vision algorithm that can be used to track multiple objects in a video. It outperforms older methods by considering all detected objects, even those with a lower confidence. The algorithm is especially useful in busy or rapidly changing environments.
While it's not perfect, especially when objects are hidden for a long time or require significant computing power for real-time processing, ByteTrack is still a very valuable asset. It can be used across many industries for use cases, including autonomous driving, manufacturing, and more. If you're a developer looking to enhance your object-tracking capabilities, ByteTrack is definitely worth exploring.
Keep Reading
Want to learn more? Check out these resources:
- An article on how to track and count using YOLOv8
- A guide on how to track objects using ByteTrack
- More on how to use ByteTrack to build computer vision applications
Cite this Post
Use the following entry to cite this post in your research:
Contributing Writer. (Aug 21, 2024). What is ByteTrack? A Deep Dive.. Roboflow Blog: https://blog.roboflow.com/what-is-bytetrack-computer-vision/
Discuss this Post
If you have any questions about this blog post, start a discussion on the Roboflow Forum.